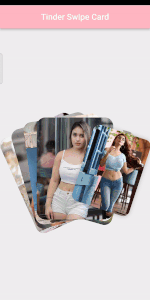
Hi guys I hope you all are doing well and also “HAPPY NEW YEAR”. So today we learn and create a tinder swipe card in a flutter. this type of card is used in images and information in a swipe on the left side or right side per user requirements. you usually see on tinder and other dating sites or you can implement this card into every app as per your requirements. In this article, we explain you to how to use it, where to implement and how to create your UI attractive to the users. Now move to the coding part.
Implementing a Tinder-like nice swipe card feature using flutter is quite easy because of the powerful widget provided by Flutter SDK. In this article, I’m going a show you how to implement the swipe card function in Flutter using several known widgets.
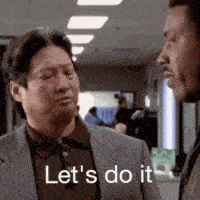
Step 1: First you implement the tinder swipe card package
First you open your pubspec.yaml file and implement swipe_deck 1.1.0 package into your project
dependencies: swipe_deck: ^1.1.0
Step 2: Open your Main.dart file
Open your Main.dart file and add this code like this
import 'package:flutter/material.dart'; import 'package:flutter/services.dart'; import 'TinderSwipeCard/tinder_swip_card.dart'; void main() { WidgetsFlutterBinding.ensureInitialized(); SystemChrome.setEnabledSystemUIMode(SystemUiMode.immersiveSticky, overlays:[]).then( (_) => runApp(MyApp()), ); // runApp(MyApp()); } class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return new MaterialApp( debugShowCheckedModeBanner: false, home: TinderSwipeCard(), ); } }
I am using SystemChrome.setEnabledSystemUIMode() class which is hidden bottom navigation bar and upper notification bar also. and inside this class, we add runApp(MyApp()) which means whole entire app hides the bottom navigation bar and top notification bar this is a cool trick please try this.
Step 3: create and open your Tinder.dart file and implement this code
Open your Tinder.dart file and add this code to your file. In this code, you create an array list at the top of the code and add the image file name which you add to the assets folder the minimum array size is 4 images. in your choice of how many images to add to this array which indicates the card list which is created the final result.
import 'package:flutter/material.dart'; import 'package:swipe_deck/swipe_deck.dart'; const IMAGES = ["tinder_card1", "tinder_card2","tinder_card3","tinder_card4","tinder_card5","tinder_card6","tinder_card7","tinder_card8","tinder_card9","tinder_card10","tinder_card11","tinder_card12","tinder_card13","tinder_card14","tinder_card15","tinder_card16","tinder_card17","tinder_card18","tinder_card19","tinder_card20","tinder_card21","tinder_card22","tinder_card23","tinder_card24",]; final borderRadius = BorderRadius.circular(20.0); class TinderSwipeCard extends StatefulWidget { const TinderSwipeCard({Key? key}) : super(key: key); @override State<TinderSwipeCard> createState() => _TinderSwipeCardState(); } class _TinderSwipeCardState extends State<TinderSwipeCard> { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text("Tinder Swipe Card"), centerTitle: true, backgroundColor: Color(0xFFffb6c1), ), body: Center( child: Container( color: Color(0xFFefefef), width: 400, child: Center( child: SwipeDeck( startIndex: 3, emptyIndicator: Container( child: Center( child: Text("Nothing Here"), ), ), cardSpreadInDegrees: 8, // Change the Spread of Background Cards onSwipeLeft: (){ print("USER SWIPED LEFT -> GOING TO NEXT WIDGET"); }, onSwipeRight: (){ print("USER SWIPED RIGHT -> GOING TO PREVIOUS WIDGET"); }, onChange: (index){ print(IMAGES[index]); }, widgets: IMAGES .map((e) => GestureDetector( onTap: () { print(e); }, child: ClipRRect( borderRadius: borderRadius, child: Image.asset( "assets/images/$e.jpg", fit: BoxFit.cover, )), )) .toList(), ), ), ), ), ); } }
Output
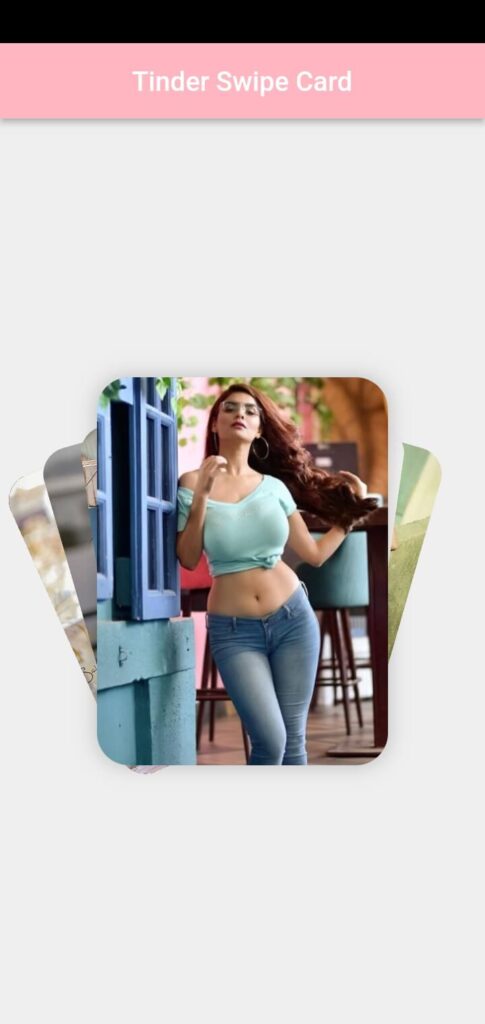
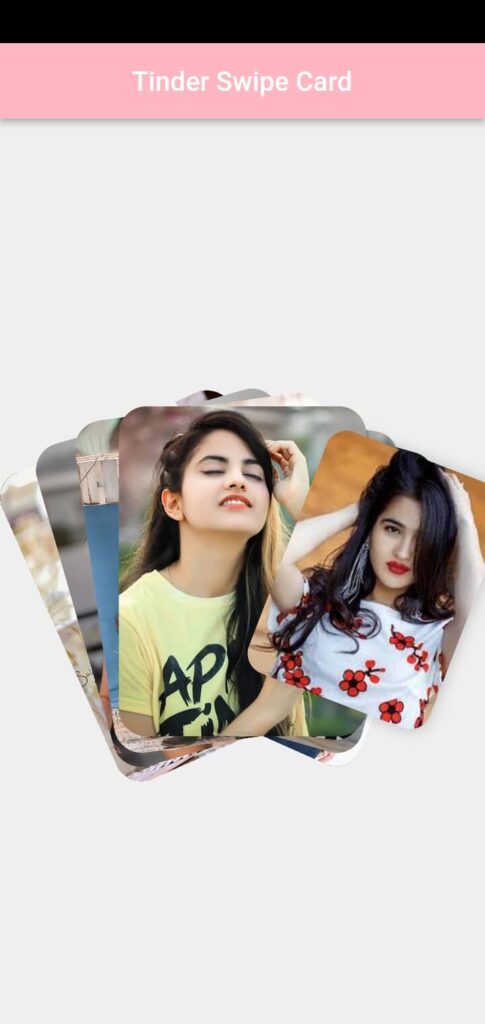
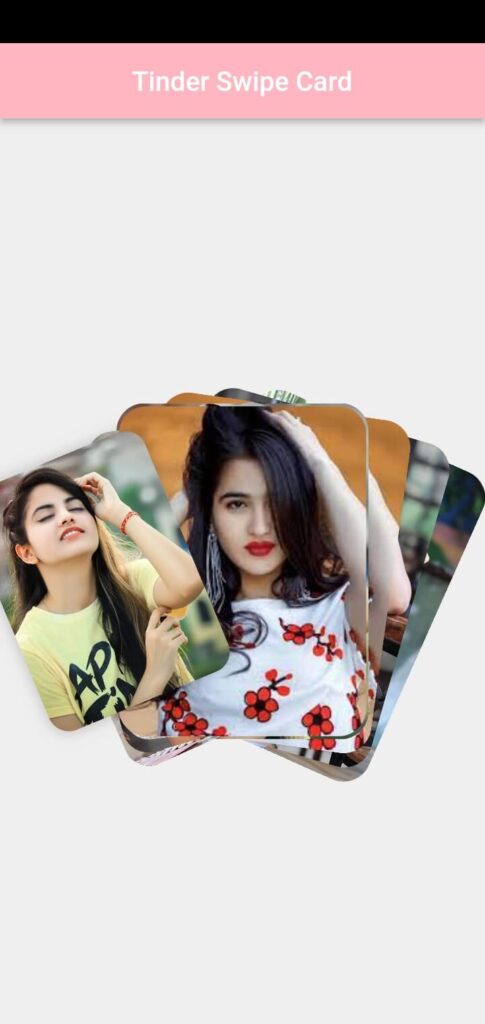
Conclusion
in this article, we create an attractive and beautiful tinder swipe card with the help of swipe_cards: ^1.0.0 package which is so useful and easy to implement. Implementing a Tinder-like nice swipe card feature using flutter is quite easy because of the powerful widget provided by Flutter SDK. In this article, I’m going a show you how to implement the swipe card function in Flutter using several known widgets.
Thanks for reading this article ❤
If I got something wrong? Let me know in the comments. I would love to improve.
Clap 👏 If this article helps you.
If we got something wrong? Let me know in the comments. we would love to improve.
And if you like my work then you also check our more interesting articles: How to implement Curved Navigation bar into Flutter, How to implement Login Interactive Form with Rive Animation, How to Implementing Navigation Drawer Animation into Flutter