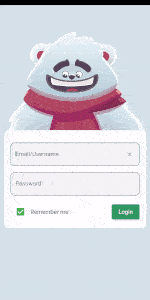
Hi, guys I hope you all are doing well. So today we create a most demanding and attractive Login form with Rive Animation in flutter. Rive Animation is a new format for real-time animation, with a dedicated editor and runtimes to play your content on all major platforms. Though we’re working to solve a bigger problem, Rive already does all the things Lottie can do and more. Now first, we understand what is rive animation.
Rive Animation in flutter is solving a bigger problem
Rive is solving a different problem compared to After Effects (Lottie’s editor) and other design tools. Is rive better to Lottie or why rive animation is best? Yes, since However, Lottie uses the runtime-incompatible After Effects format, which was never intended for use. As a result, Rive is not only far more capable than Lottie but also smaller, quicker, and more efficient. There’s a new category that has been missing; a design tool whose primary purpose is to build content that is interactive and meant to ship in products. Not just mock-ups, prototypes, or linear videos.
Most graphics nowadays run in real-time interactive software, yet our design tools are built to export static graphics or linear animations. There needs to be a tool that exports an interactive format that can run anywhere. Like png, jpg, or mp4, but interactive and non-linear. I figured everyone would have a lot of questions about how to use rive animation in Flutter, how to make rive animation in flutter. I’m now clearing up all of your doubts and moving on to the coding portion.
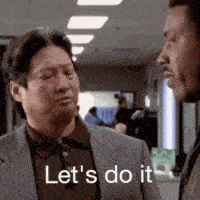
Step 1: First open the Rive Animation site and create an account
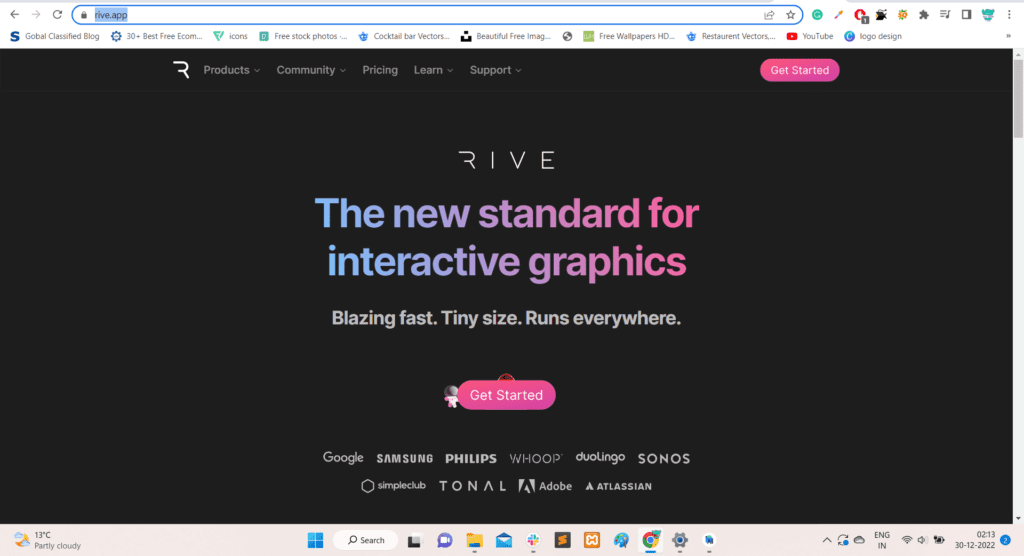
If you want attractive animation then you open a Rive animation official site and create an account. then click to get started button and explore and create a new animation. but don’t worry I’m sharing the file click the link: Click to download the file.
Step 2: Implement the Rive package in your Flutter project
Open your pubspec.yaml file and implement the rive 0.10.0 package into your project.
dependencies: rive: ^0.10.0
Step 3: Open your main.dart file
Open your main.dart file and put this code into your file
import 'package:flutter/material.dart'; import 'package:flutter/services.dart'; import 'RivLogin/riv_login.dart'; void main() { WidgetsFlutterBinding.ensureInitialized(); SystemChrome.setEnabledSystemUIMode(SystemUiMode.immersiveSticky, overlays:[]).then( (_) => runApp(MyApp()), ); // runApp(MyApp()); } class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return new MaterialApp( debugShowCheckedModeBanner: false, home: LoginForm(), ); } }
Step 4: Open your created class LoginForm.dart file
Open your LoginForm.dart file and implement this code into your file I am explaining the code after this
import 'package:flutter/foundation.dart'; import 'package:flutter/material.dart'; import 'package:flutter/services.dart'; import 'package:motion_toast/motion_toast.dart'; import 'package:motion_toast/resources/arrays.dart'; import 'package:rive/rive.dart'; class LoginForm extends StatefulWidget { const LoginForm({Key? key}) : super(key: key); @override State<LoginForm> createState() => _LoginFormState(); } class _LoginFormState extends State<LoginForm> { late String animationURL; Artboard? _teddyArtboard; SMITrigger? successTrigger, failTrigger; SMIBool? isHandsUp, isChecking; SMINumber? numLook; bool checkBoxValue = true; StateMachineController? stateMachineController; final TextEditingController _emailController = TextEditingController(); final TextEditingController _passwordController = TextEditingController(); @override void initState() { // TODO: implement initState super.initState(); animationURL = defaultTargetPlatform == TargetPlatform.android || defaultTargetPlatform == TargetPlatform.iOS ? 'assets/animation/login.riv' : 'animations/login.riv'; rootBundle.load(animationURL).then( (data) { final file = RiveFile.import(data); final artboard = file.mainArtboard; stateMachineController = StateMachineController.fromArtboard(artboard, "Login Machine"); if (stateMachineController != null) { artboard.addController(stateMachineController!); stateMachineController!.inputs.forEach((e) { debugPrint(e.runtimeType.toString()); debugPrint("name${e.name}End"); }); stateMachineController!.inputs.forEach((element) { if (element.name == "trigSuccess") { successTrigger = element as SMITrigger; } else if (element.name == "trigFail") { failTrigger = element as SMITrigger; } else if (element.name == "isHandsUp") { isHandsUp = element as SMIBool; } else if (element.name == "isChecking") { isChecking = element as SMIBool; } else if (element.name == "numLook") { numLook = element as SMINumber; } }); } setState(() => _teddyArtboard = artboard); }, ); } void handsOnTheEyes() { isHandsUp?.change(true); } void lookOnTheTextField() { isHandsUp?.change(false); isChecking?.change(true); numLook?.change(0); } void moveEyeBalls(val) { numLook?.change(val.length.toDouble()); } void login() { isChecking?.change(false); isHandsUp?.change(false); if (_emailController.text == "[email protected]" && _passwordController.text == "admin") { isChecking?.change(false); isHandsUp?.change(false); successTrigger?.fire(); _displaySuccessToast(); } else { isChecking?.change(false); isHandsUp?.change(false); failTrigger?.fire(); _displayErrorToast(); } } void _displayErrorToast() { MotionToast.error( title: const Text( 'Error', style: TextStyle( fontWeight: FontWeight.bold, ), ), description: const Text('Please enter correct name and password'), position: MotionToastPosition.bottom, barrierColor: Colors.black.withOpacity(0.3), width: 300, height: 80, dismissable: true, ).show(context); } void _displaySuccessToast() { MotionToast.success( title: const Text( 'Sucess', style: TextStyle( fontWeight: FontWeight.bold, ), ), description: const Text('email & password are correct'), position: MotionToastPosition.bottom, barrierColor: Colors.black.withOpacity(0.3), width: 300, height: 80, dismissable: true, ).show(context); } @override Widget build(BuildContext context) { return Scaffold( backgroundColor: const Color(0xffd6e2ea), body: SingleChildScrollView( child: Center( child: Column( mainAxisAlignment: MainAxisAlignment.center, children: [ if (_teddyArtboard != null) SizedBox( width: 400, height: 300, child: Rive( artboard: _teddyArtboard!, fit: BoxFit.fitWidth, ), ), Container( alignment: Alignment.center, width: 400, padding: EdgeInsets.only(bottom: 15), margin: EdgeInsets.only(bottom: 15 * 4, left: 10, right: 10), decoration: BoxDecoration( color: Colors.white, borderRadius: BorderRadius.circular(10), ), child: Column( children: [ Padding( padding: const EdgeInsets.symmetric(horizontal: 15), child: Column( children: [ const SizedBox(height: 15 * 2), TextField( controller: _emailController, onTap: lookOnTheTextField, onChanged: moveEyeBalls, keyboardType: TextInputType.emailAddress, enableSuggestions: false, autocorrect: false, style: const TextStyle(fontSize: 14), cursorColor: const Color(0xff319964), decoration: InputDecoration( hintText: "Email/Username", suffixIcon: IconButton( icon: Icon(Icons.close,color: Colors.grey,size: 18,), onPressed: () => _emailController.clear(), ), filled: true, border: OutlineInputBorder( borderRadius: BorderRadius.all(Radius.circular(10)), ), focusColor: Color(0xff319964), focusedBorder: OutlineInputBorder( borderSide: BorderSide( color: Color(0xff319964), ), borderRadius: BorderRadius.all(Radius.circular(10)), ), ), ), const SizedBox(height: 15), TextField( controller: _passwordController, onTap: handsOnTheEyes, keyboardType: TextInputType.visiblePassword, obscureText: true, style: const TextStyle(fontSize: 14), cursorColor: const Color(0xffb04863), decoration: const InputDecoration( hintText: "Password", filled: true, border: OutlineInputBorder( borderRadius: BorderRadius.all(Radius.circular(10)), ), focusColor: Color(0xffb04863), focusedBorder: OutlineInputBorder( borderSide: BorderSide( color: Color(0xffb04863), ), borderRadius: BorderRadius.all(Radius.circular(10)), ), ), ), const SizedBox(height: 15), Row( crossAxisAlignment: CrossAxisAlignment.end, mainAxisAlignment: MainAxisAlignment.spaceBetween, children: [ //remember me checkbox Row( children: [ Checkbox( value: checkBoxValue, activeColor: Colors.green, onChanged: (newvalue) { setState(() { checkBoxValue = newvalue!; }); }, ), Text("Remember me"), ], ), ElevatedButton( onPressed: login, style: ElevatedButton.styleFrom( backgroundColor: const Color(0xff319964), ), child: Text("Login"), ), ], ), ], ), ), ], ), ), ], ), ), ), ); } }
Output
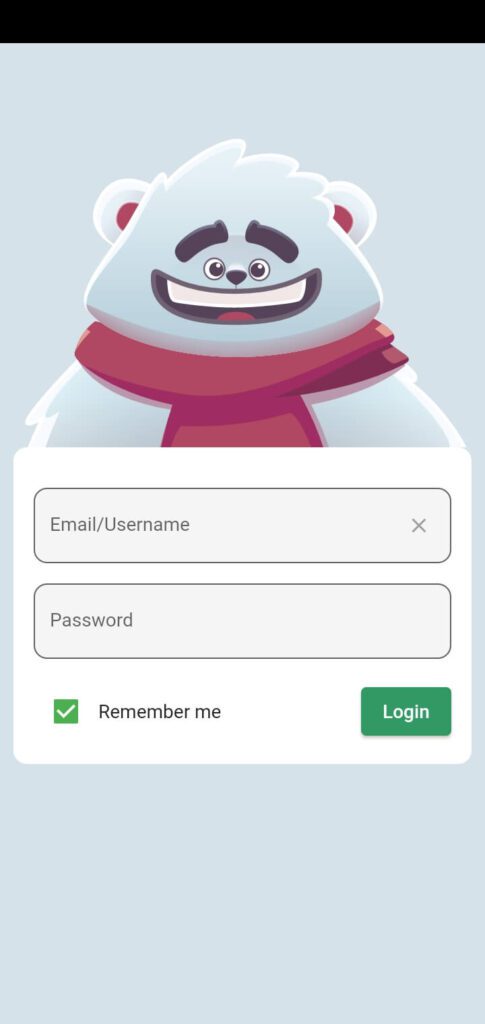
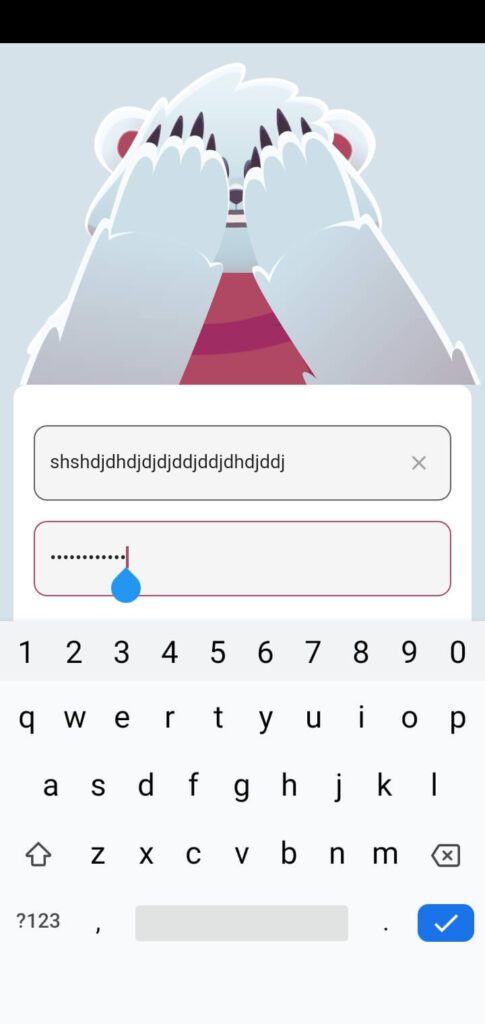
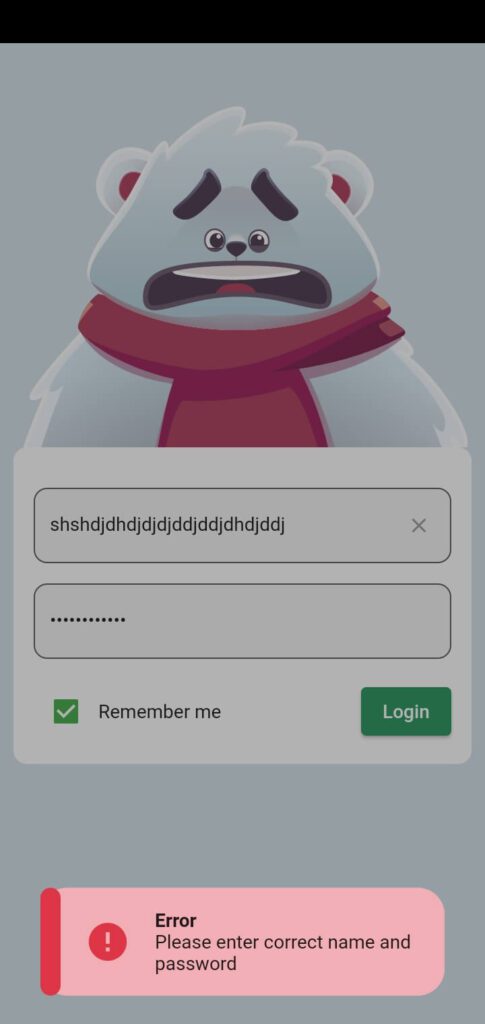
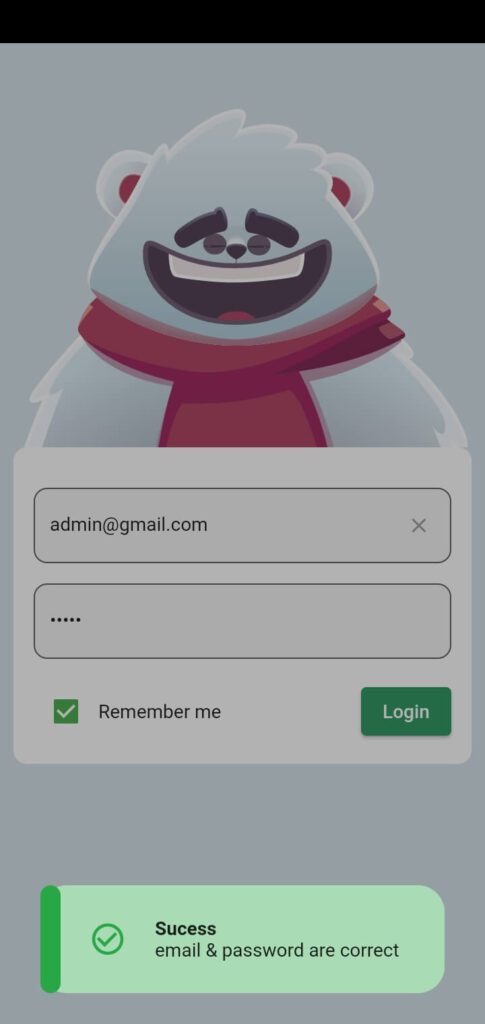
Conclusion
In this article, we create a beautiful & attractive Login form that attracts users into the user app and makes them more eyes catching. we implement the Rive Animation package into our app to access the animation functionality. Then you create an account on the riv.app site and create and explore a new animation and then download and implement it into our app.
Thanks for reading this article ❤
If I got something wrong? Let me know in the comments. I would love to improve.
Clap 👏 If this article helps you.
If you got something wrong? Let me know in the comments. we would love to improve.
and if you like my work then you also check our new attractive articles like How to Implement Showcase view in a flutter, How to create a Scratch Card in flutter using a scratcher package, How to create Toast Notification in a flutter.