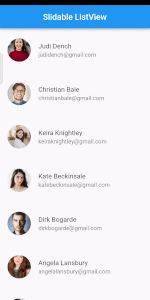
Hi, guys I hope you all are doing well So in this article we learn to create and implement Slidable ListView into our flutter apps. First, we understand that is a ListView; ListView is a very important widget in flutter apps. It is used to create a list of children but when we want to create a list without writing code again and again then ListView.builder is used instead of ListView. ListView.builder Creates a scrollable, linear array of widgets. Now we understand what is a Slidable ListView, where we use Slidable ListView, and why we use this.
Slidable ListView
Slidable ListView in an application can be utilized to play out a wide scope of tasks with simply a straightforward swipe to one or the other right or left on the tile. It makes the UI very easy to understand and recovers a ton of time doing trifling tasks that can be riotous and repetitive to tasks whenever done in alternate manners.
Features
- Accepts start (left/top) and end (right/bottom) action panes.
- Can be dismissed.
- 4 built-in action panes.
- 2 built-in slide action widgets.
- 1 built-in dismiss animation.
- You can easily create custom layouts and animations.
- You can use a builder to create your slide actions if you want special effects during animation.
- Closes when a slide action has been tapped (overridable).
- Closes when the nearest
Scrollable
starts to scroll (overridable). - Option to disable the slide effect easily.
Now I think you understand what is ListView & Slidable ListView. So now move to the coding part.
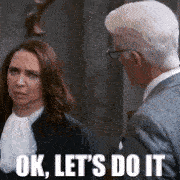
Step 1: First we implement Slidable ListView package
Open your pubspec.yaml file and implement flutter_slidable 2.0.0 and put this code and click the Pub get button.
dependencies: flutter_slidable: ^2.0.0
Step 2: Open your main.dart file
Open your Main.dart file and add this code like this
import 'package:flutter/material.dart'; import 'package:flutter/services.dart'; import 'SlidableListView/slidable_listView.dart'; void main() { WidgetsFlutterBinding.ensureInitialized(); SystemChrome.setEnabledSystemUIMode(SystemUiMode.immersiveSticky, overlays:[]).then( (_) => runApp(MyApp()), ); // runApp(MyApp()); } class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return new MaterialApp( debugShowCheckedModeBanner: false, home: SlidableListView(), ); } }
I am using SystemChrome.setEnabledSystemUIMode() class which is hidden bottom navigation bar and upper notification bar also. and inside this class, we add runApp(MyApp()) which means whole entire app hides the bottom navigation bar and top notification bar this is a cool trick please try this.
Step 3: Open & Create SlidableListView.dart class
Open your SlidableListView.dart file and implement simple Scaffold Widget like this
import 'package:flutter/material.dart'; class SlidableListView extends StatefulWidget { const SlidableListView({Key? key}) : super(key: key); @override State<SlidableListView> createState() => _SlidableListViewState(); } class _SlidableListViewState extends State<SlidableListView> { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text('Slidable ListView'), centerTitle: true, ), body: Container() ); } }
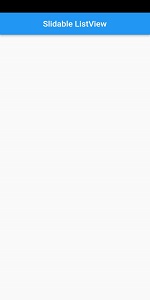
Step 4: Create one Model class User.dart
Create a User.dart file for storing local data in it you can also class from the API
class User{ final String name; final String email; final String image; User(this.name, this.email, this.image); } final allusers = [ User("Judi Dench", "[email protected]", "https://images.unsplash.com/photo-1494790108377-be9c29b29330?ixlib=rb-4.0.3&ixid=MnwxMjA3fDB8MHxzZWFyY2h8MXx8dXNlciUyMHByb2ZpbGV8ZW58MHx8MHx8&w=1000&q=80"), User("Christian Bale", "[email protected]", "https://us.123rf.com/450wm/lacheev/lacheev2109/lacheev210900016/lacheev210900016.jpg?ver=6"), User("Keira Knightley", "[email protected]", "https://us.123rf.com/450wm/deagreez/deagreez2104/deagreez210409586/deagreez210409586.jpg?ver=6"), User("Kate Beckinsale", "[email protected]", "https://www.rd.com/wp-content/uploads/2017/09/01-shutterstock_476340928-Irina-Bg.jpg?fit=640,427"), User("Dirk Bogarde", "[email protected]", "https://encrypted-tbn0.gstatic.com/images?q=tbn:ANd9GcSPSX6rnIejKF6jwH56EVGfSiimk09IYi1JAIYmSIf4Z__hXs-jP8RHKlbVc4GhSVyM_Ns&usqp=CAU"), User("Angela Lansbury", "[email protected]", "https://i.pinimg.com/564x/6b/28/21/6b2821072600f08832f4af96e965273b--narnia-brunettes.jpg"), User("Robert Carlyle", "[email protected]", "https://images.pexels.com/photos/2379005/pexels-photo-2379005.jpeg?cs=srgb&dl=pexels-italo-melo-2379005.jpg&fm=jpg"), User("Thandiwe Newton", "[email protected]", "https://images.unsplash.com/photo-1502323703385-c3ea9ace787d?ixlib=rb-4.0.3&ixid=MnwxMjA3fDB8MHxwaG90by1yZWxhdGVkfDR8fHxlbnwwfHx8fA%3D%3D&w=1000&q=80"), User("Sacha Baron Cohen", "[email protected]", "https://images.pexels.com/photos/2379004/pexels-photo-2379004.jpeg?auto=compress&cs=tinysrgb&dpr=1&w=500"), User("Olivia Colman", "[email protected]", "https://t4.ftcdn.net/jpg/01/15/85/23/360_F_115852367_E6iIYA8OxHDmRhjw7kOq4uYe4t440f14.jpg"), ];
Step 5: Open your SlidableListView.dart file and implement Slidable()
Slidable( startActionPane: ActionPane( motion: StretchMotion(), children: [ SlidableAction( backgroundColor: Colors.green, icon: Icons.share, label: 'Share', onPressed: (context)=> _onShareArchived(index,Actions.share), ), SlidableAction( backgroundColor: Colors.blue, icon: Icons.archive, label: 'Archive', onPressed: (context)=> _onShareArchived(index,Actions.archive), ) ], ), endActionPane: ActionPane( motion: BehindMotion(), dismissible: DismissiblePane( onDismissed: ()=>_onDismissed(index, Actions.delete), ), children: [ SlidableAction( backgroundColor: Colors.red, icon: Icons.delete, label: 'Delete', onPressed: (context)=> _onDismissed(index,Actions.delete), ) ], ), child: buildUserListTile(user));
Step 6: Full code and implement for Slidable ListView in SlidableListView.dart file
import 'package:flutter/material.dart'; import 'package:flutter_slidable/flutter_slidable.dart'; import 'package:navigationdrawer/SlidableListView/user.dart'; enum Actions{share,delete,archive} class SlidableListView extends StatefulWidget { const SlidableListView({Key? key}) : super(key: key); @override State<SlidableListView> createState() => _SlidableListViewState(); } class _SlidableListViewState extends State<SlidableListView> { List<User> users = allusers; @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text('Slidable ListView'), centerTitle: true, ), body: SlidableAutoCloseBehavior( closeWhenOpened: true, child: ListView.builder( itemCount: users.length, itemBuilder: (context, index) { final user = users[index]; return Slidable( startActionPane: ActionPane( motion: StretchMotion(), children: [ SlidableAction( backgroundColor: Colors.green, icon: Icons.share, label: 'Share', onPressed: (context)=> _onShareArchived(index,Actions.share), ), SlidableAction( backgroundColor: Colors.blue, icon: Icons.archive, label: 'Archive', onPressed: (context)=> _onShareArchived(index,Actions.archive), ) ], ), endActionPane: ActionPane( motion: BehindMotion(), dismissible: DismissiblePane( onDismissed: ()=>_onDismissed(index, Actions.delete), ), children: [ SlidableAction( backgroundColor: Colors.red, icon: Icons.delete, label: 'Delete', onPressed: (context)=> _onDismissed(index,Actions.delete), ) ], ), child: buildUserListTile(user)); }), ), ); } void _onShareArchived(int index, Actions action){ final user = users[index]; switch (action){ case Actions.archive: _showSnackBar(context, '${user.name} is archived', Colors.blue,Duration(seconds: 1)); break; case Actions.share: _showSnackBar(context, '${user.name} is shared', Colors.green,Duration(seconds: 1)); break; } } void _onDismissed(int index, Actions action){ final user = users[index]; setState(() { users.removeAt(index); }); _showSnackBar(context, '${user.name} is deleted', Colors.red,Duration(seconds: 1)); } void _showSnackBar(BuildContext context, String message, Color color,Duration duration){ final snackBar = SnackBar(content: Text(message),backgroundColor: color,duration: duration,); ScaffoldMessenger.of(context).showSnackBar(snackBar); } Widget buildUserListTile(User user) => Builder( builder: (context)=> ListTile( contentPadding: EdgeInsets.all(16), title: Text(user.name), subtitle: Text(user.email), leading: CircleAvatar( radius: 30, backgroundImage: NetworkImage(user.image), ), onTap: (){ final slidable = Slidable.of(context); final isClosed = slidable?.actionPaneType.value == ActionPaneType.none; if(isClosed){ slidable?.openStartActionPane(); }else{ slidable?.close(); } }, ), ); }
Output
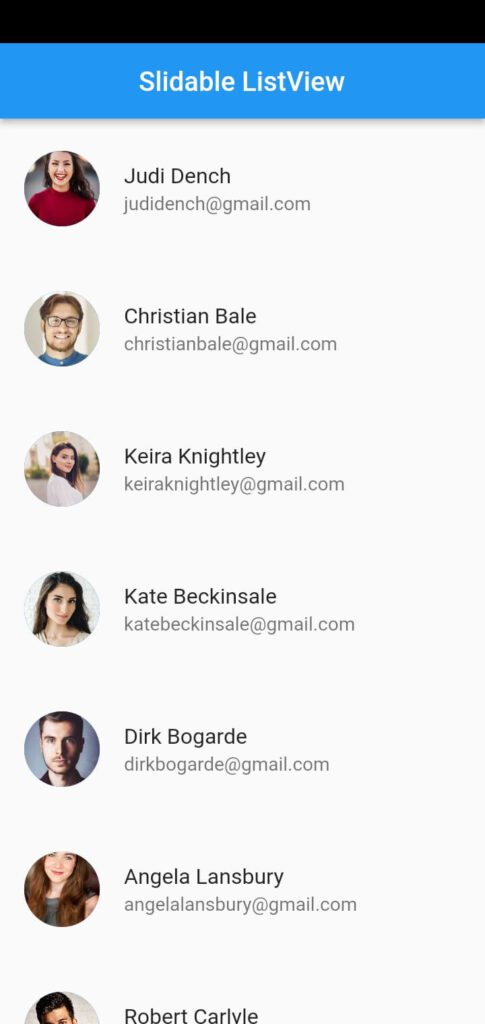
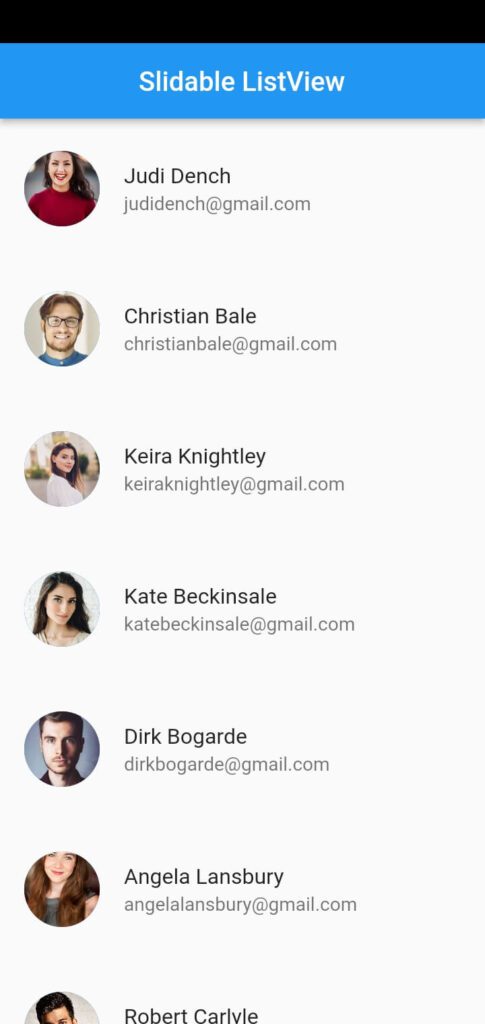
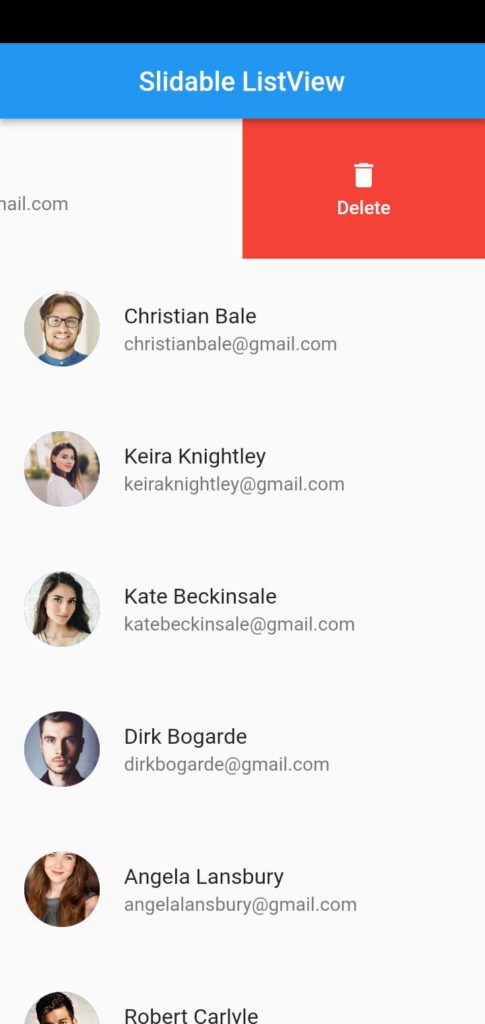
Conclusion
So in this article, we show how to create a slidable listview in a flutter and show how slidable will work when swiped left to right the tile and when swiped right to left the tile in your flutter applications using flutter_slidable package, and then they will be shown on your device.
I hope this article will provide you with sufficient information in Trying up Slidable ListView in your flutter projects. We will show you what is slidable, some features used in slidable, make a demo program for working slidable, and show when swiped left to right archives and share option on the tile and when the swiped right to left deletes and more options on the tiles in your flutter applications, So please try it.
❤ ❤ Thanks for reading this article ❤❤
If you like my work and want some more interesting and attractive articles also read this: How to implement tinder swipe card in a flutter, How to Implement Showcase view in a flutter, How to implement Login Interactive Form with Rive Animation.