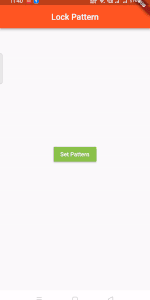
Hi guys I hope you doing well. So today we learn, create and implement a Pattern lock screen into our Flutter project. In this article, we will explore the Pattern lock Screen In Flutter. We learn how to implement a demo program lock screen using the pattern_lock 2.0.0 package in your flutter applications. First, we know what is a pattern lock and where and why we use it. We use pattern lock to help secure your Android phone or tablet. Each time you turn on your device or wake up the screen, you’ll be asked to unlock your device, usually with a PIN, pattern, or password. On some devices, you can unlock it with your fingerprint. and this functionality we use to save and secure our files also. Now we go to the coding part and implement step by step.
Step 1: First we implement a Pattern lock screen into our project
Open your pubspec.yaml file and implement pattern_lock 2.0.0 like this
dependencies: pattern_lock: ^2.0.0
Step 2: Open your Main.dart file
import 'package:flutter/material.dart'; import 'LockPattern/LockPatternPage.dart'; void main() { runApp(MyApp()); } class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return new MaterialApp(home: new LockPatternPage()); } }
Step3: Create and open LockPatternPage.dart file
import 'package:flutter/material.dart'; import 'package:navigationdrawer/LockPattern/util.dart'; import 'CheckPattern.dart'; import 'SetPattern.dart'; class LockPatternPage extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( title: 'Pattern Lock Demo', theme: ThemeData( primarySwatch: Colors.deepOrange, ), routes: { "/check_pattern": (BuildContext context) => CheckPattern(), "/set_pattern": (BuildContext context) => SetPattern(), "/big_pattern": (BuildContext context) => BigPattern(), }, home: Scaffold( appBar: AppBar( title: Text('Lock Pattern'), centerTitle: true, ), body: LockPatternMain(), ), ); } } class LockPatternMain extends StatefulWidget { @override _LockPatternMainWidgetState createState() => _LockPatternMainWidgetState(); } class _LockPatternMainWidgetState extends State<LockPatternMain> { List<int>? pattern; @override Widget build(BuildContext context) { return Center( child: Column( crossAxisAlignment: CrossAxisAlignment.center, mainAxisAlignment: MainAxisAlignment.center, children: <Widget>[ MaterialButton( color: Colors.lightGreen, child: Text("Set Pattern", style: TextStyle(color: Colors.white)), onPressed: () async { final result = await Navigator.pushNamed(context, "/set_pattern"); if (result is List<int>) { context.replaceSnackbar( content: Text("pattern is $result",textAlign: TextAlign.center,style: TextStyle(fontSize: 12,fontWeight: FontWeight.bold,color: Colors.white),), color: Colors.deepOrange, ); setState(() { pattern = result; }); } }, ), if (pattern != null) ...[ SizedBox(height: 16), MaterialButton( color: Colors.green, child: Text("Check Pattern", style: TextStyle(color: Colors.white),textAlign: TextAlign.center,), onPressed: () async { final result = await Navigator.pushNamed( context, "/check_pattern", arguments: pattern, ); if (result == true) { context.replaceSnackbar( content: Text("it's correct",textAlign: TextAlign.center, style: TextStyle(color: Colors.white,fontSize: 12,), ), color: Colors.green, ); } }, ), ], ], ), ); } }
Step 4: Create SetPattern, CheckPattern, util.dart file like this
import 'package:flutter/foundation.dart'; import 'package:flutter/material.dart'; import 'package:navigationdrawer/LockPattern/util.dart'; import 'package:pattern_lock/pattern_lock.dart'; class SetPattern extends StatefulWidget { @override _SetPatternState createState() => _SetPatternState(); } class _SetPatternState extends State<SetPattern> { bool isConfirm = false; List<int>? pattern; final scaffoldKey = GlobalKey<ScaffoldState>(); @override Widget build(BuildContext context) { return Scaffold( key: scaffoldKey, appBar: AppBar( title: Text("Check Pattern"), ), body: Column( mainAxisAlignment: MainAxisAlignment.spaceEvenly, children: <Widget>[ Flexible( child: Text( isConfirm ? "Confirm pattern" : "Draw pattern", style: TextStyle(fontSize: 26), ), ), Flexible( child: PatternLock( selectedColor: Colors.amber, pointRadius: 12, onInputComplete: (List<int> input) { if (input.length < 3) { context.replaceSnackbar( content: Text("At least 3 points required", textAlign: TextAlign.center, style: TextStyle(color: Colors.white,fontSize: 12), ), color: Colors.red, ); return; } if (isConfirm) { if (listEquals<int>(input, pattern)) { Navigator.of(context).pop(pattern); } else { context.replaceSnackbar( content: Text("Patterns do not match", textAlign: TextAlign.center, style: TextStyle(color: Colors.white,fontSize: 12), ), color: Colors.red, ); setState(() { pattern = null; isConfirm = false; }); } } else { setState(() { pattern = input; isConfirm = true; }); } }, ), ), ], ), ); } }
import 'package:flutter/foundation.dart'; import 'package:flutter/material.dart'; import 'package:navigationdrawer/LockPattern/util.dart'; import 'package:pattern_lock/pattern_lock.dart'; class CheckPattern extends StatelessWidget { final scaffoldKey = GlobalKey<ScaffoldState>(); @override Widget build(BuildContext context) { final List<int>? pattern = ModalRoute.of(context)!.settings.arguments as List<int>?; return Scaffold( key: scaffoldKey, appBar: AppBar( title: Text("Check Pattern"), ), body: Column( mainAxisAlignment: MainAxisAlignment.spaceEvenly, children: <Widget>[ Flexible( child: Text( "Draw Your pattern", style: TextStyle(fontSize: 26), ), ), Flexible( child: PatternLock( selectedColor: Colors.red, pointRadius: 8, showInput: true, dimension: 3, relativePadding: 0.7, selectThreshold: 25, fillPoints: true, onInputComplete: (List<int> input) { if (listEquals<int>(input, pattern)) { Navigator.of(context).pop(true); } else { context.replaceSnackbar( content: Text("wrong", textAlign: TextAlign.center, style: TextStyle(color: Colors.white,fontSize: 12), ), color: Colors.red, ); } }, ), ), ], ), ); } }
import 'package:flutter/foundation.dart'; import 'package:flutter/material.dart'; import 'package:navigationdrawer/LockPattern/util.dart'; import 'package:pattern_lock/pattern_lock.dart'; class CheckPattern extends StatelessWidget { final scaffoldKey = GlobalKey<ScaffoldState>(); @override Widget build(BuildContext context) { final List<int>? pattern = ModalRoute.of(context)!.settings.arguments as List<int>?; return Scaffold( key: scaffoldKey, appBar: AppBar( title: Text("Check Pattern"), ), body: Column( mainAxisAlignment: MainAxisAlignment.spaceEvenly, children: <Widget>[ Flexible( child: Text( "Draw Your pattern", style: TextStyle(fontSize: 26), ), ), Flexible( child: PatternLock( selectedColor: Colors.red, pointRadius: 8, showInput: true, dimension: 3, relativePadding: 0.7, selectThreshold: 25, fillPoints: true, onInputComplete: (List<int> input) { if (listEquals<int>(input, pattern)) { Navigator.of(context).pop(true); } else { context.replaceSnackbar( content: Text("wrong", textAlign: TextAlign.center, style: TextStyle(color: Colors.white,fontSize: 12), ), color: Colors.red, ); } }, ), ), ], ), ); } }
Implement all the packages in all files and run your app and output like this
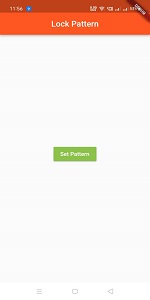
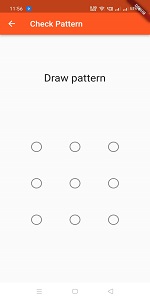
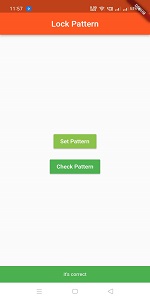
Conclusion
In the article, I have explained the Pattern lock screen basic structure in a flutter; you can modify this code according to your choice. This was a small introduction to the Pattern lock screen On User Interaction from my side, and it’s working using Flutter.
I hope this blog will provide you with sufficient information in Trying up the Pattern lock screen in your flutter projects. We will show you what the Pattern lock screen is? So please try it.
❤ ❤ Thanks for reading this article ❤❤
If I got something wrong? Let me know in the comments. I would love to improve.
Clap 👏 If this article helps you.
If you like our work so you can also read these amazing articles; How to implement a Foldable Navigation Sidebar on Flutter, How To Hide Bottom Navigation Bar On Scroll in Flutter, How to implement ListWheelScrollView in Flutter