Before starting our blog let’s quickly access what you will load on your memory after reading this blog.
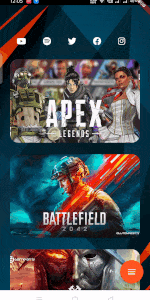
Hi guys I hope you all doing well. Today we create an amazing and Interesting Foldable Navigation Sidebar on our Flutter project. this is used when you attract the user to your app and grab users’ attention with the content. So don’t waste your time First we understand how to build this SideBar. it is so easy to use and customize.
Step 1: First add Foldable Navigation Sidebar package into your project
you need to add foldable_sidebar: ^1.0.0 package into your pubspec.yaml file .
dependencies: foldable_sidebar: ^1.0.0
Step 2: Add this code to main.dart file
add this simple code into your main.dart file.
import 'package:flutter/material.dart'; import 'package:foldable_sidebar/foldable_sidebar.dart'; void main() { runApp(MyApp()); } class MyApp extends StatelessWidget { // This widget is the root of your application. @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', theme: ThemeData( primarySwatch: Colors.blue, visualDensity: VisualDensity.adaptivePlatformDensity, ), home: MyHomePage(), ); } } class MyHomePage extends StatefulWidget { @override _MyHomePageState createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { FSBStatus drawerStatus; @override Widget build(BuildContext context) { return SafeArea( child: Scaffold( body: FoldableSidebarBuilder( drawerBackgroundColor: Colors.deepOrange, drawer: CustomDrawer(closeDrawer: (){ setState(() { drawerStatus = FSBStatus.FSB_CLOSE; }); },), screenContents: FirstScreen(), status: drawerStatus, ), floatingActionButton: FloatingActionButton( backgroundColor: Colors.deepOrange, child: Icon(Icons.menu,color: Colors.white,), onPressed: () { setState(() { drawerStatus = drawerStatus == FSBStatus.FSB_OPEN ? FSBStatus.FSB_CLOSE : FSBStatus.FSB_OPEN; }); }), ), ); } } class FirstScreen extends StatelessWidget { @override Widget build(BuildContext context) { return Container( color: Colors.black.withAlpha(200), child: Center(child: Text("Click on FAB to Open Drawer",style: TextStyle(fontSize: 20,color: Colors.white),),), ); } } class CustomDrawer extends StatelessWidget { final Function closeDrawer; const CustomDrawer({Key key, this.closeDrawer}) : super(key: key); @override Widget build(BuildContext context) { MediaQueryData mediaQuery = MediaQuery.of(context); return Container( color: Colors.white, width: mediaQuery.size.width * 0.60, height: mediaQuery.size.height, child: Column( children: <Widget>[ Container( width: double.infinity, height: 200, child: Image.network( "https://png.pngtree.com/thumb_back/fh260/back_pic/02/50/63/71577e1cf59d802.jpg", width: double.infinity, height: 174, fit: BoxFit.cover, ), ), ListTile( onTap: (){ debugPrint("Tapped Profile"); }, leading: Icon(Icons.person,color: Colors.black,size: 30,), title: Text( "Your Profile", style: TextStyle(fontWeight: FontWeight.bold,color: Colors.black), ), ), Divider( height: 1, color: Colors.grey, ), ListTile( onTap: () { debugPrint("Tapped settings"); }, leading: Icon(Icons.settings,size: 30,color: Colors.black,), title: Text("Settings", style: TextStyle(fontWeight: FontWeight.bold,color: Colors.black), ), ), Divider( height: 1, color: Colors.grey, ), ListTile( onTap: () { debugPrint("Tapped Payments"); }, leading: Icon(Icons.payment,size: 30,color: Colors.black,), title: Text("Payments", style: TextStyle(fontWeight: FontWeight.bold,color: Colors.black),), ), Divider( height: 1, color: Colors.grey, ), ListTile( onTap: () { debugPrint("Tapped Notifications"); }, leading: Icon(Icons.notifications,size: 30,color: Colors.black,), title: Text("Notifications", style: TextStyle(fontWeight: FontWeight.bold,color: Colors.black),), ), Divider( height: 1, color: Colors.grey, ), ListTile( onTap: () { debugPrint("Tapped Log Out"); }, leading: Icon(Icons.exit_to_app,size: 30,color: Colors.black,), title: Text("Log Out", style: TextStyle(fontWeight: FontWeight.bold,color: Colors.black),), ), ], ), ); } }
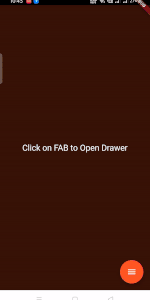
If you want to open sidebar with the swipe to right and clode to left then you add this pacakge and code.
Add Swipedetector package into pubspec.yaml flie
dependencies: swipedetector: ^1.2.0
import 'package:flutter/material.dart'; import 'package:foldable_sidebar/foldable_sidebar.dart'; import 'package:swipedetector/swipedetector.dart'; void main() { runApp(MyApp()); } class MyApp extends StatelessWidget { // This widget is the root of your application. @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', theme: ThemeData( primarySwatch: Colors.blue, visualDensity: VisualDensity.adaptivePlatformDensity, ), home: MyHomePage(), ); } } class MyHomePage extends StatefulWidget { @override _MyHomePageState createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { FSBStatus drawerStatus; @override Widget build(BuildContext context) { return SafeArea( child: Scaffold( body: SwipeDetector( onSwipeRight: (){ setState(() { drawerStatus = FSBStatus.FSB_OPEN; }); }, onSwipeLeft: (){ drawerStatus = FSBStatus.FSB_CLOSE; }, child: FoldableSidebarBuilder( drawerBackgroundColor: Colors.deepOrange, drawer: CustomDrawer(closeDrawer: (){ setState(() { drawerStatus = FSBStatus.FSB_CLOSE; }); },), screenContents: FirstScreen(), status: drawerStatus, ), ), floatingActionButton: FloatingActionButton( backgroundColor: Colors.deepOrange, child: Icon(Icons.menu,color: Colors.white,), onPressed: () { setState(() { drawerStatus = drawerStatus == FSBStatus.FSB_OPEN ? FSBStatus.FSB_CLOSE : FSBStatus.FSB_OPEN; }); }), ), ); } } class FirstScreen extends StatelessWidget { @override Widget build(BuildContext context) { return Container( color: Colors.black.withAlpha(200), child: Center(child: Text("Click on FAB to Open Drawer",style: TextStyle(fontSize: 20,color: Colors.white),),), ); } } class CustomDrawer extends StatelessWidget { final Function closeDrawer; const CustomDrawer({Key key, this.closeDrawer}) : super(key: key); @override Widget build(BuildContext context) { MediaQueryData mediaQuery = MediaQuery.of(context); return Container( color: Colors.white, width: mediaQuery.size.width * 0.60, height: mediaQuery.size.height, child: Column( children: <Widget>[ Container( width: double.infinity, height: 200, child: Image.network( "https://png.pngtree.com/thumb_back/fh260/back_pic/02/50/63/71577e1cf59d802.jpg", width: double.infinity, height: 174, fit: BoxFit.cover, ), ), ListTile( onTap: (){ debugPrint("Tapped Profile"); }, leading: Icon(Icons.person,color: Colors.black,size: 30,), title: Text( "Your Profile", style: TextStyle(fontWeight: FontWeight.bold,color: Colors.black), ), ), Divider( height: 1, color: Colors.grey, ), ListTile( onTap: () { debugPrint("Tapped settings"); }, leading: Icon(Icons.settings,size: 30,color: Colors.black,), title: Text("Settings", style: TextStyle(fontWeight: FontWeight.bold,color: Colors.black), ), ), Divider( height: 1, color: Colors.grey, ), ListTile( onTap: () { debugPrint("Tapped Payments"); }, leading: Icon(Icons.payment,size: 30,color: Colors.black,), title: Text("Payments", style: TextStyle(fontWeight: FontWeight.bold,color: Colors.black),), ), Divider( height: 1, color: Colors.grey, ), ListTile( onTap: () { debugPrint("Tapped Notifications"); }, leading: Icon(Icons.notifications,size: 30,color: Colors.black,), title: Text("Notifications", style: TextStyle(fontWeight: FontWeight.bold,color: Colors.black),), ), Divider( height: 1, color: Colors.grey, ), ListTile( onTap: () { debugPrint("Tapped Log Out"); }, leading: Icon(Icons.exit_to_app,size: 30,color: Colors.black,), title: Text("Log Out", style: TextStyle(fontWeight: FontWeight.bold,color: Colors.black),), ), ], ), ); } }
If you facing some errors like null safety then you remove null safety into your project like open your pubspec.yaml file then remove sdk enviroment
Before
environment: sdk: ">=2.16.2 <3.0.0"
After
environment: sdk: '>=2.11.0 <3.0.0'
Now if you want the attractive and interesting Design then you add this code into your project and easy to customize.
import 'package:flutter/material.dart'; import 'package:foldable_sidebar/foldable_sidebar.dart'; import 'package:font_awesome_flutter/font_awesome_flutter.dart'; import 'package:swipedetector/swipedetector.dart'; void main() { runApp(MyApp()); } class MyApp extends StatelessWidget { // This widget is the root of your application. @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', theme: ThemeData( primarySwatch: Colors.blue, visualDensity: VisualDensity.adaptivePlatformDensity, ), home: MyHomePage(), ); } } class MyHomePage extends StatefulWidget { @override _MyHomePageState createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { FSBStatus drawerStatus; @override Widget build(BuildContext context) { return SafeArea( child: Scaffold( body: SwipeDetector( onSwipeRight: (){ setState(() { drawerStatus = FSBStatus.FSB_OPEN; }); }, onSwipeLeft: (){ drawerStatus = FSBStatus.FSB_CLOSE; }, child: FoldableSidebarBuilder( drawerBackgroundColor: Colors.deepOrange, drawer: CustomDrawer(closeDrawer: (){ setState(() { drawerStatus = FSBStatus.FSB_CLOSE; }); },), screenContents: FirstScreen(), status: drawerStatus, ), ), floatingActionButton: FloatingActionButton( backgroundColor: Colors.deepOrange, child: Icon(Icons.menu,color: Colors.white,), onPressed: () { setState(() { drawerStatus = drawerStatus == FSBStatus.FSB_OPEN ? FSBStatus.FSB_CLOSE : FSBStatus.FSB_OPEN; }); }), ), ); } } class FirstScreen extends StatelessWidget { @override Widget build(BuildContext context) { return Stack( children: <Widget>[ Image.network( "https://static.vecteezy.com/system/resources/previews/001/849/553/original/modern-gold-background-free-vector.jpg", height: MediaQuery.of(context).size.height, width: MediaQuery.of(context).size.width, fit: BoxFit.cover, ), Scaffold( backgroundColor: Colors.transparent, appBar: AppBar( backgroundColor: Colors.transparent, elevation: 0.0, ), body: SingleChildScrollView( child: Column( children: <Widget>[ Padding( padding: const EdgeInsets.all(20.0), child: Row( children: <Widget>[ Padding( padding: const EdgeInsets.only(left: 20,right: 20), child: FaIcon(FontAwesomeIcons.youtube,color: Colors.white,size: 20,), ), Padding( padding: const EdgeInsets.only(left: 20,right: 20), child: FaIcon(FontAwesomeIcons.spotify,color: Colors.white,size: 20,), ), Padding( padding: const EdgeInsets.only(left: 20,right: 20), child: FaIcon(FontAwesomeIcons.twitter,color: Colors.white,size: 20,), ), Padding( padding: const EdgeInsets.only(left: 20,right: 20), child: FaIcon(FontAwesomeIcons.facebook,color: Colors.white,size: 20,), ), Padding( padding: const EdgeInsets.only(left: 20,right: 20), child: FaIcon(FontAwesomeIcons.instagram,color: Colors.white,size: 20,), ), ], ), ), Padding( padding: const EdgeInsets.only(left: 20,right: 20,top: 10,bottom: 10), child: Card( shape: RoundedRectangleBorder( borderRadius: BorderRadius.circular(20.0), ), child: Column( children: <Widget>[ Container( height: 200, decoration: BoxDecoration( image: DecorationImage( image: NetworkImage('https://9to5fortnite.com/wp-content/uploads/2022/03/apex-legends-pick-rate-most-popular-legends.jpg'), fit: BoxFit.cover ), borderRadius: BorderRadius.circular(20.0), ), ), ], ), ), ), Padding( padding: const EdgeInsets.only(left: 20,right: 20,top: 10,bottom: 10), child: Card( shape: RoundedRectangleBorder( borderRadius: BorderRadius.circular(20.0), ), child: Column( children: <Widget>[ Container( height: 200, decoration: BoxDecoration( image: DecorationImage( image: NetworkImage('https://wp.clutchpoints.com/wp-content/uploads/2021/06/Battlefield-2042-ditches-single-player-mode-will-be-strictly-multiplayer.jpg'), fit: BoxFit.cover ), borderRadius: BorderRadius.circular(20.0), ), ), ], ), ), ), Padding( padding: const EdgeInsets.only(left: 20,right: 20,top: 10,bottom: 10), child: Card( shape: RoundedRectangleBorder( borderRadius: BorderRadius.circular(20.0), ), child: Column( children: <Widget>[ Container( height: 200, decoration: BoxDecoration( image: DecorationImage( image: NetworkImage('https://i.ytimg.com/vi/cz1iX4ME-m0/maxresdefault.jpg'), fit: BoxFit.cover ), borderRadius: BorderRadius.circular(20.0), ), ), ], ), ), ), Padding( padding: const EdgeInsets.only(left: 20,right: 20,top: 10,bottom: 10), child: Card( shadowColor: Colors.grey, shape: RoundedRectangleBorder( borderRadius: BorderRadius.circular(20.0), ), child: Column( children: <Widget>[ Container( height: 200, decoration: BoxDecoration( image: DecorationImage( image: NetworkImage('https://images.ctfassets.net/umhrp0op95v1/7rn68TUGN1lZuQRQz1zqSY/f3da676f0ce14f07653cfc5a97aad211/la-key-600.jpg'), fit: BoxFit.cover ), borderRadius: BorderRadius.circular(20.0), ), ), ], ), ), ), Padding( padding: const EdgeInsets.only(left: 20,right: 20,top: 10,bottom: 10), child: Card( shadowColor: Colors.grey, shape: RoundedRectangleBorder( borderRadius: BorderRadius.circular(20.0), ), child: Column( children: <Widget>[ Container( height: 200, decoration: BoxDecoration( image: DecorationImage( image: NetworkImage('https://playidlegames.com/wp-content/uploads/2019/12/10-advanced-tips-guide-for-mastering-free-fire.jpg'), fit: BoxFit.cover ), borderRadius: BorderRadius.circular(20.0), ), ), ], ), ), ), ], ), ) ) ], ); } } class CustomDrawer extends StatelessWidget { final Function closeDrawer; const CustomDrawer({Key key, this.closeDrawer}) : super(key: key); @override Widget build(BuildContext context) { MediaQueryData mediaQuery = MediaQuery.of(context); return Container( width: mediaQuery.size.width * 0.60, height: mediaQuery.size.height, decoration: const BoxDecoration( image: DecorationImage( image: NetworkImage("https://img.freepik.com/free-vector/hand-painted-watercolor-pastel-sky-background_23-2148902771.jpg?w=2000"), fit: BoxFit.cover), ), child: Column( children: <Widget>[ SizedBox(height: 20,), Container( width: double.infinity, height: 100, color: Colors.grey.withAlpha(20), child: CircleAvatar( backgroundImage: NetworkImage("https://i.pinimg.com/originals/72/3d/0a/723d0af616b1fe7d5c7e56a3532be3cd.png"), ) ), ListTile( onTap: (){ debugPrint("Tapped Profile"); }, leading: Icon(Icons.person_pin,color: Colors.white,size: 30,), title: Text( "Your Profile", ), ), Divider( height: 1, color: Colors.grey, ), ListTile( onTap: () { debugPrint("Tapped settings"); }, leading: Icon(Icons.settings_phone,color: Colors.white,size: 30,), title: Text("Settings"), ), Divider( height: 1, color: Colors.grey, ), ListTile( onTap: () { debugPrint("Tapped Payments"); }, leading: Icon(Icons.payment,color: Colors.white,size: 30,), title: Text("Payments"), ), Divider( height: 1, color: Colors.grey, ), ListTile( onTap: () { debugPrint("Tapped Notifications"); }, leading: Icon(Icons.notifications,color: Colors.white,size: 30,), title: Text("Notifications"), ), Divider( height: 1, color: Colors.grey, ), ListTile( onTap: () { debugPrint("Tapped Log Out"); }, leading: Icon(Icons.exit_to_app,color: Colors.white,size: 30,), title: Text("Log Out"), ), ], ), ); } }
Output
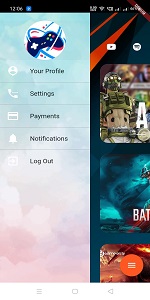
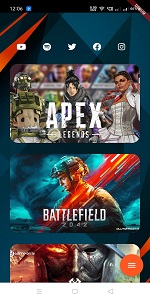