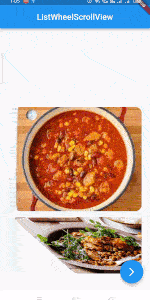
Hi guys I hope you doing well in your life. So today we learn How to implement ListWheelScrollView in Flutter app. First, we understand what is ListView, ListView is a scrollable list of widgets arranged linearly. It displays its children one after the other in the scroll direction i.e. vertical or horizontal. Now we move to ListWheelScrollView. Listwheelscrollview A box in which children on wheels can be scrolled. This widget is similar to ListView but with the restriction that all children must be the same size along the scrolling axis.
Now here are some properties of ListWheelScrollView:
- childDelegate: This property takes ListWheelChildDelegate class as the parameter value (final). It lazily initiates the child.
- childBehaviour: This property takes Clip enum as the parameter. It controls the portion of the content to be clipped.
- controller: This property takes ScrollController class as the parameter and it is used to control the current item.
- diameterRatio: This property takes a double value as the parameter and it determines the ratio between the size of the wheel and the size of vireport.
- itemExtent: This property takes a double value as the parameter, which has to be a positive number. It decides the size of each child in the wheel.
- magnification: This property also takes in a double value as the parameter and zoom-in value, by default it is set to 1.0. If it is greater than 1.0 the center element will be zoomed by that factor and vice-versa.
- OffAxisFraction: This property also takes in a double value as the parameter and controls how the wheel is horizontally off-center.
I thought you understand what is ListWheelScrollView now moving to the Code part. Let’s Start.
How to implement ListWheelScrollView in Flutter
import 'package:flutter/material.dart'; class ListWheelScrollViewPage extends StatefulWidget { const ListWheelScrollViewPage({Key? key}) : super(key: key); @override State<ListWheelScrollViewPage> createState() => _ListWheelScrollViewPageState(); } class _ListWheelScrollViewPageState extends State<ListWheelScrollViewPage> { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text('ListWheelScrollView'), centerTitle: true, ), body: Padding( padding: EdgeInsets.all(20.0), child: ListWheelScrollView( itemExtent: 280, children: [ Container( height: 100, decoration: BoxDecoration( color: Color(0xFF319964), borderRadius: BorderRadius.circular(15), ), child: Center( child: Text('Item 1', textAlign: TextAlign.center, style: TextStyle( color: Colors.white, fontSize: 50.0, ), ), ), ), Container( height: 100, decoration: BoxDecoration( color: Colors.blue, borderRadius: BorderRadius.circular(15), ), child: Center( child: Text('Item 2', textAlign: TextAlign.center, style: TextStyle( color: Colors.white, fontSize: 50.0, ), ), ), ), Container( height: 100, decoration: BoxDecoration( color: Colors.red, borderRadius: BorderRadius.circular(15), ), child: Center( child: Text('Item 3', textAlign: TextAlign.center, style: TextStyle( color: Colors.white, fontSize: 50.0, ), ), ), ), Container( height: 100, decoration: BoxDecoration( color: Colors.yellow, borderRadius: BorderRadius.circular(15), ), child: Center( child: Text('Item 4', textAlign: TextAlign.center, style: TextStyle( color: Colors.white, fontSize: 50.0, ), ), ), ), ], ), ) ); } }
Output

As you can see our single container inside the list is assigned to the middle because of the ListWheelScrollView . The selected child is displayed in the center or front of your screen. As you can see we have added several containers inside the Children property of the ListWheelScrollView class and this gives a more 3-D view look to our list. now it’s time to see a more interesting property of this widget and try to implement them and create an interesting and attractive UI too.
import 'package:flutter/cupertino.dart'; import 'package:flutter/material.dart'; class ListWheelScrollViewPage extends StatefulWidget { const ListWheelScrollViewPage({Key? key}) : super(key: key); @override State<ListWheelScrollViewPage> createState() => _ListWheelScrollViewPageState(); } class _ListWheelScrollViewPageState extends State<ListWheelScrollViewPage> { late FixedExtentScrollController controller; @override void initState() { // TODO: implement initState super.initState(); controller = FixedExtentScrollController(); } @override void dispose() { controller.dispose(); super.dispose(); } @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text('ListWheelScrollView'), centerTitle: true, ), floatingActionButton: FloatingActionButton( child: Icon(Icons.arrow_forward_ios_outlined), onPressed: (){ final nextIndex = controller.selectedItem + 1; controller.animateToItem( nextIndex, duration: Duration(seconds: 1), curve: Curves.easeInOut, ); }, ), body: Padding( padding: EdgeInsets.all(20.0), child: ListWheelScrollView( controller: controller, itemExtent: 250, physics: FixedExtentScrollPhysics(), offAxisFraction: -1.5, children: [ Container( height: 100, decoration: BoxDecoration( borderRadius: BorderRadius.circular(15), image: DecorationImage( image: AssetImage('assets/DraggableImages/draggle1.jpg'), fit: BoxFit.cover ) ), ), Container( height: 100, decoration: BoxDecoration( borderRadius: BorderRadius.circular(15), image: DecorationImage( image: AssetImage('assets/DraggableImages/draggle2.jpg'), fit: BoxFit.cover ) ), ), Container( height: 100, decoration: BoxDecoration( borderRadius: BorderRadius.circular(15), image: DecorationImage( image: AssetImage('assets/DraggableImages/draggle3.jpg'), fit: BoxFit.cover ) ), ), Container( height: 100, decoration: BoxDecoration( borderRadius: BorderRadius.circular(15), image: DecorationImage( image: AssetImage('assets/DraggableImages/draggle4.jpg'), fit: BoxFit.cover ) ), ), Container( height: 100, decoration: BoxDecoration( borderRadius: BorderRadius.circular(15), image: DecorationImage( image: AssetImage('assets/DraggableImages/draggle5.jpg'), fit: BoxFit.cover ) ), ), Container( height: 100, decoration: BoxDecoration( borderRadius: BorderRadius.circular(15), image: DecorationImage( image: AssetImage('assets/DraggableImages/draggle6.jpg'), fit: BoxFit.cover ) ), ), Container( height: 100, decoration: BoxDecoration( borderRadius: BorderRadius.circular(15), image: DecorationImage( image: AssetImage('assets/DraggableImages/draggle7.jpg'), fit: BoxFit.cover ) ), ), Container( height: 100, decoration: BoxDecoration( borderRadius: BorderRadius.circular(15), image: DecorationImage( image: AssetImage('assets/DraggableImages/draggle8.jpg'), fit: BoxFit.cover ) ), ), Container( height: 100, decoration: BoxDecoration( borderRadius: BorderRadius.circular(15), image: DecorationImage( image: AssetImage('assets/DraggableImages/draggle9.jpg'), fit: BoxFit.cover ) ), ), Container( height: 100, decoration: BoxDecoration( borderRadius: BorderRadius.circular(15), image: DecorationImage( image: AssetImage('assets/DraggableImages/draggle10.jpg'), fit: BoxFit.cover ) ), ), ], ), ) ); } }
Output
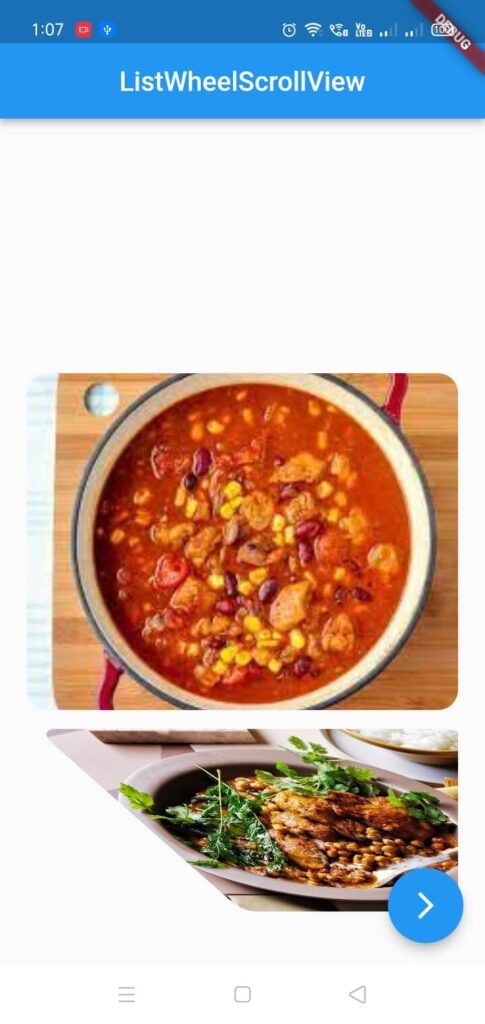
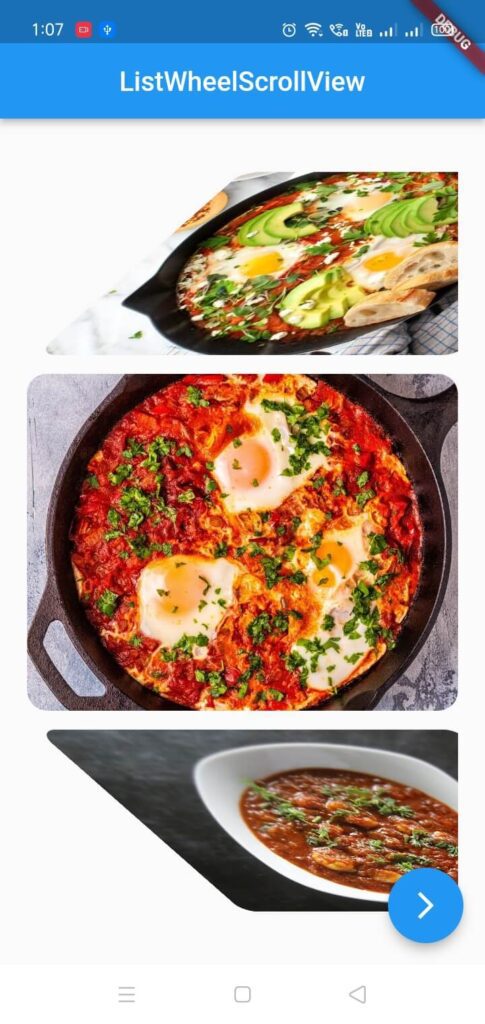
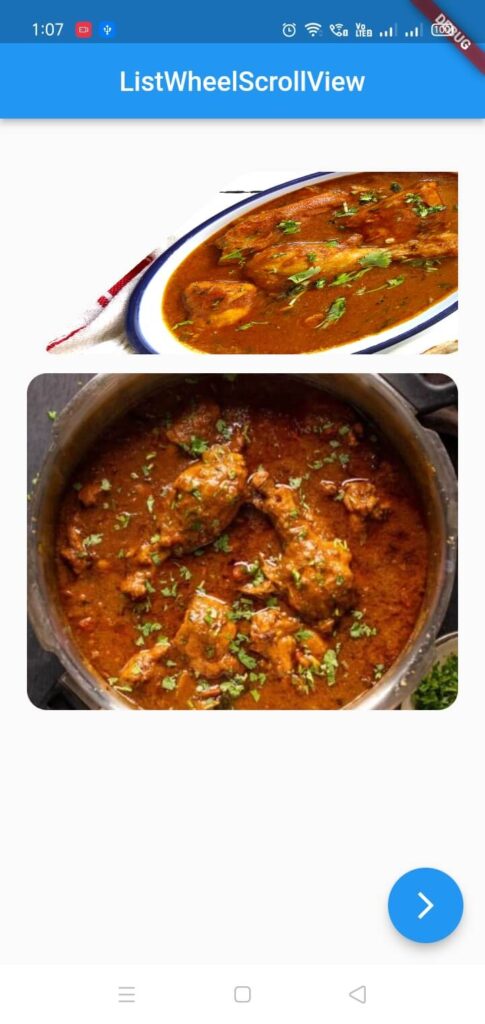