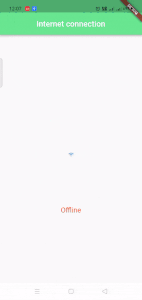
Hi, guys so today we learn how to check the internet connection in a flutter, and also we create separate define both of them. While developing any flutter app, you might want to check the internet connection or not connected status. Maybe you wanted to do it before when you hit API call or listing to the internet connection status to let users know about it proactively. To check the internet connection on flutter, you need to add connectivity plus plugin and check the internet connection manually and it’s called checkConnection method and also listen to whether the network connectivity change or not by calling onConnectivityChnage.listen method. So in this tutorial, we’ll see the step-by-step guide the implementing Flutter internet connection checker.
Step 1: First we add the Connectivity plus package in our project for internet connection in a flutter
dependencies:
connectivity_plus: ^3.0.2
Step 2: Create a class name NetworkConnectivity.dart to check network connectivity
import 'dart:async';
import 'dart:io';
import 'package:connectivity_plus/connectivity_plus.dart';
class NetworkConnectivity {
NetworkConnectivity._();
static final _instance = NetworkConnectivity._();
static NetworkConnectivity get instance => _instance;
final _networkConnectivity = Connectivity();
final _controller = StreamController.broadcast();
Stream get myStream => _controller.stream;
void initialise() async {
ConnectivityResult result = await _networkConnectivity.checkConnectivity();
_checkStatus(result);
_networkConnectivity.onConnectivityChanged.listen((result) {
print(result);
_checkStatus(result);
});
}
void _checkStatus(ConnectivityResult result) async {
bool isOnline = false;
try {
final result = await InternetAddress.lookup('google.com');
isOnline = result.isNotEmpty && result[0].rawAddress.isNotEmpty;
} on SocketException catch (_) {
isOnline = false;
}
_controller.sink.add({result: isOnline});
}
void disposeStream() => _controller.close();
}
Step 3: Implementing this code in your main file for internet connection in a flutter, in my case is ConnectionCheckerDemo.dart
import 'package:connectivity_plus/connectivity_plus.dart';
import 'package:flutter/cupertino.dart';
import 'package:flutter/foundation.dart';
import 'package:flutter/material.dart';
import 'package:lottie/lottie.dart';
import 'NetworkConnectivity.dart';
class ConnectionCheckerDemo extends StatefulWidget {
const ConnectionCheckerDemo({Key? key}) : super(key: key);
@override
State<ConnectionCheckerDemo> createState() => _ConnectionCheckerDemoState();
}
class _ConnectionCheckerDemoState extends State<ConnectionCheckerDemo> {
Map _source = {ConnectivityResult.none: false};
final NetworkConnectivity _networkConnectivity = NetworkConnectivity.instance;
var string = "";
@override
void initState() {
super.initState();
_networkConnectivity.initialise();
_networkConnectivity.myStream.listen((source) {
_source = source;
print('source $_source');
// 1.
switch (_source.keys.toList()[0]) {
case ConnectivityResult.mobile:
string =
_source.values.toList()[0] ? 'Online' : 'Offline';
break;
case ConnectivityResult.wifi:
string =
_source.values.toList()[0] ? 'Online' : 'Offline';
break;
case ConnectivityResult.none:
default:
string = 'Offline';
}
// 2.
setState(() {});
// 3.
string == "Online" ?
ScaffoldMessenger.of(context).showSnackBar(
SnackBar(
content: Text("ONLINE", style: TextStyle(fontSize: 14),textAlign: TextAlign.center,),
backgroundColor: Colors.green,
),
) : ScaffoldMessenger.of(context).showSnackBar(
SnackBar(
content: Text("OFFLINE", style: TextStyle(fontSize: 10),textAlign: TextAlign.center,),
backgroundColor: Colors.red,
duration: Duration(seconds: 5),
),
);
});
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
centerTitle: true,
title: Text('Internet connection'),
backgroundColor: const Color(0xff6ae792),
),
body: Center(
child: string == 'Online' ?
Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Lottie.network('https://assets8.lottiefiles.com/packages/lf20_fjv8qxqn.json'),
Text("Online", style: TextStyle(fontSize: 18,color: Colors.green),),
],
) : Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Lottie.network('https://assets3.lottiefiles.com/packages/lf20_ibgol5nq.json'),
Text("Offline", style: TextStyle(fontSize: 18,color: Colors.red),),
],
)
),
);
}
@override
void dispose() {
_networkConnectivity.disposeStream();
super.dispose();
}
}
Output
Conclusion
in this tutorial, we learn how to implement an internet connection in a flutter with practical examples. For this tutorial, we use the connectivity plus plugin and used its methods to check the internet connection and also we use the Lottie package for attractive UI. We are just calling a lookup function with the passing string “google.com”. If the result is not empty we call the setstate() function, and change the variable Active Connection to true, other false, we saw both the approach of checking the internet connection manually and listening to the connectivity status change automatically.
Would you like to check other interesting Flutter tutorials? like how to implement Day Night Time Picker in Flutter, How to create Onboarding Screen Sliders on Flutter, and How to implement Date Picker in a flutter.