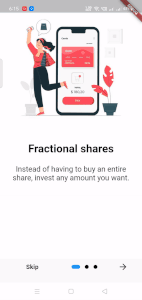
Hii guys in this article we learn how to create apps Onboarding screens with the help of the introduction screen Flutter package. The onboarding screen is the best way to introduce your app to the users. but the design, screen, and animation are very hard for beginners. Here we explained to make the introduction screen easy with very less codes.
In our example, we have the following structure:
- assets/
- —— images/
- ————– img1.png
- ————– img2.png
- ————– img3.png
- lib/
- —— main.dart
- —— intro_screen.dart
- —— home.dart
- pubspec.yaml
Step 1: Add the introduction_screen Flutter package in your dependency and enable the assets folder in your pubspec.yaml and also add images also inside the assets folders.
dependencies:
flutter:
sdk: flutter
introduction_screen: ^1.0.8
assets:
- assets/image1.jpg
- assets/image2.jpg
- assets/image3.jpg
- assets/image4.jpg
Step 2: Add this code to navigate to your onboarding screen in main.dart file.
import 'package:flutter/material.dart';
import 'package:navigationdrawer/Page2.dart';
class BottomNavigation extends StatefulWidget {
BottomNavigation ({Key? key}) : super(key: key);
@override
_MyNavigationBarState createState() => _MyNavigationBarState();
}
class _MyNavigationBarState extends State<BottomNavigation > {
@override
Widget build(BuildContext context) {
return Scaffold(
body: IntroScreen()
);
}
}
Step 3: Implement this code into your IntroScreen.dart file.
import 'package:flutter/material.dart';
import 'package:introduction_screen/introduction_screen.dart';
import 'package:navigationdrawer/HomePage.dart';
class IntroScreen extends StatefulWidget{
@override
State<StatefulWidget> createState() {
return _IntroScreen();
}
}
class _IntroScreen extends State<IntroScreen>{
@override
Widget build(BuildContext context) {
//this is a page decoration for intro screen
PageDecoration pageDecoration = PageDecoration(
titleTextStyle: TextStyle(fontSize: 28.0,
fontWeight: FontWeight.w700,
color:Colors.black
), //tile font size, weight and color
bodyTextStyle: TextStyle(fontSize: 19.0, color:Colors.black),
//body text size and color
// descriptionPadding: EdgeInsets.fromLTRB(16.0, 0.0, 16.0, 16.0),
//decription padding
imagePadding: EdgeInsets.all(20), //image padding
boxDecoration:BoxDecoration(
color: Colors.white
), //show linear gradient background of page
);
return IntroductionScreen(
//main background of screen
pages: [ //set your page view here
PageViewModel(
title: "Fractional shares",
body: "Instead of having to buy an entire share, invest any amount you want.",
image: introImage('assets/image1.jpg'),
decoration: pageDecoration,
),
PageViewModel(
title: "Fractional shares",
body: "Instead of having to buy an entire share, invest any amount you want.",
image: introImage('assets/image2.jpg'),
decoration: pageDecoration,
),
PageViewModel(
title: "Fractional shares",
body: "Instead of having to buy an entire share, invest any amount you want.",
image: introImage('assets/image3.jpg'),
decoration: pageDecoration,
),
//add more screen here
],
onDone: () => goHomepage(context), //go to home page on done
onSkip: () => goHomepage(context), // You can override on skip
showSkipButton: true,
// skipFlex: 0,
nextFlex: 0,
skip: Text('Skip', style: TextStyle(color: Colors.black,fontSize: 16,fontWeight: FontWeight.bold),),
next: Icon(Icons.arrow_forward, color: Colors.black,size: 25,),
done: Text('Getting Stated', style: TextStyle(
fontWeight: FontWeight.bold, color:Colors.black,fontSize: 16
),),
dotsDecorator: const DotsDecorator(
size: Size(10.0, 10.0), //size of dots
color: Colors.black, //color of dots
activeSize: Size(22.0, 10.0),
//activeColor: Colors.white, //color of active dot
activeShape: RoundedRectangleBorder( //shave of active dot
borderRadius: BorderRadius.all(Radius.circular(25.0)),
),
),
);
}
void goHomepage(context){
Navigator.of(context).pushAndRemoveUntil(
MaterialPageRoute(builder: (context){
return HomePage();
}
), (Route<dynamic> route) => false);
//Navigate to home page and remove the intro screen history
//so that "Back" button wont work.
}
Widget introImage(String assetName) {
//widget to show intro image
return Align(
child: Image.asset('$assetName', width: 350.0),
alignment: Alignment.bottomCenter,
);
}
}