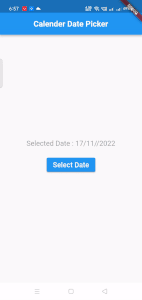
Hi guys, in this article we are creating a dynamic date range picker with simple steps. we are using showDatePicker functions that are used to select single or multiple dates along with the range between two dates. This library is so useful when we using to get dates, weeks, months, and years, and navigation between dates and even centuries is simple and easy.
The following are the key features of DatePicker:
1. Multiple Picker View: This feature allows the user to navigate between dates, months, years, and centuries with ease as shown below:
2. Multi-Date Picker View: It supports selecting single and multiple dates and also supports selecting a range between two dates as shown below:
3. RLT support: This feature is helpful for users who interact in a language that is written in Right to a left manner like Arabic and Hebrew as shown below:
Step 1: Create a new class and use a StatefulWidget, and extend it to an app bar and a body for getting a simple app structure as shown below:
import 'package:flutter/material.dart';
class CalenderDatePicker extends StatefulWidget {
const CalenderDatePicker({Key? key}) : super(key: key);
@override
State<CalenderDatePicker> createState() => _CalenderDatePickerState();
}
class _CalenderDatePickerState extends State<CalenderDatePicker> {
@override
Widget build(BuildContext context) {
final title = "Calender Date Picker";
final style = TextStyle(fontSize: 20,fontWeight: FontWeight.bold);
return Scaffold(
appBar: AppBar(
centerTitle: true,
title: Text(
title,style: style,
),
),
body: ,
);
}
}
Step 2: Create a date picker function and variables like this
final dateController = TextEditingController();
DateTime currentDate = DateTime.now();
String? selectedDateForBackendDeveloper;
datePicker(context) async {
DateTime? userSelectedDate = await showDatePicker(
context: context,
initialDate: currentDate,
// firstDate: DateTime(2022),
firstDate: DateTime.now(),
lastDate: DateTime(2030),
);
if (userSelectedDate == null) {
return;
} else {
setState(() {
currentDate = userSelectedDate;
selectedDateForBackendDeveloper =
"${currentDate.year}/${currentDate.month}/${currentDate.day}";
});
}
}
Complete Source Code:
import 'package:flutter/material.dart';
class CalenderDatePicker extends StatefulWidget {
const CalenderDatePicker({Key? key}) : super(key: key);
@override
State<CalenderDatePicker> createState() => _CalenderDatePickerState();
}
class _CalenderDatePickerState extends State<CalenderDatePicker> {
final dateController = TextEditingController();
DateTime currentDate = DateTime.now();
String? selectedDateForBackendDeveloper;
datePicker(context) async {
DateTime? userSelectedDate = await showDatePicker(
context: context,
initialDate: currentDate,
// firstDate: DateTime(2022),
firstDate: DateTime.now(),
lastDate: DateTime(2030),
);
if (userSelectedDate == null) {
return;
} else {
setState(() {
currentDate = userSelectedDate;
selectedDateForBackendDeveloper =
"${currentDate.year}/${currentDate.month}/${currentDate.day}";
});
}
}
@override
Widget build(BuildContext context) {
final title = "Calender Date Picker";
final style = TextStyle(fontSize: 20,fontWeight: FontWeight.bold);
return Scaffold(
appBar: AppBar(
centerTitle: true,
title: Text(
title,style: style,
),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Text(
"Selected Date : ${currentDate.day}/${currentDate.month}//${currentDate.year}",
style: TextStyle(fontSize: 18,color: Colors.grey),
),
const SizedBox(
height: 10,
),
Padding(
padding: EdgeInsets.all(10),
child: ElevatedButton(
onPressed: () {
datePicker(context);
},
child: Text('Select Date',style: TextStyle(fontSize: 18),),
),
),
],
),
),
);
}
}