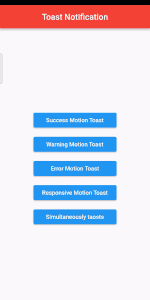
Hi guys I hope you all are doing well and preparing for the upcoming new year. So in this tutorial, we learn and create Toast Notification. We explain to create with a third-party library like fluttertoast 8.1.2. Now first we understand what is Toast Notification.
What is the toast notification in the Flutter app?
A flutter toast is a clickable, no-clickable, auto-expire element in the app that is used to display brief information in a short time. In the Android and iOS apps, it is displayed quickly and disappears after a while. The toast message is mostly used when developers show feedback on the operation performed by the users. A toast massage is one of the essential features in mobile applications that makes the app more interactive.
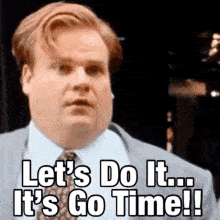
Step 1: Open your main.dart file
import 'package:flutter/material.dart'; import 'package:flutter/services.dart'; import 'package:showcaseview/showcaseview.dart'; import 'Toast/toast_notification.dart'; void main() { WidgetsFlutterBinding.ensureInitialized(); SystemChrome.setEnabledSystemUIMode(SystemUiMode.immersiveSticky, overlays:[]).then( (_) => runApp(MyApp()), ); // runApp(MyApp()); } class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return new MaterialApp( debugShowCheckedModeBanner: false, home: ShowCaseWidget( builder: Builder( builder: (_)=>ToastNotification(), ), ) ); } }
In this file, we simply navigate to ToastNotification class with the help of MaterialApp class. and debugShowCheckedModeBanner: false, which means to hide the debug icon in the top right corner.
Step 2: Open your pubspec.yaml file and implement the Toast Notification package
dependencies: fluttertoast: ^8.1.2
Open your pubspec.yaml file and put this given code and hit Pub get button and wait to import the package. and please connect with the internet connection.
Step 3: Now time to implement Toast Notification open your ToastNotification.dart
import 'package:flutter/material.dart'; import 'package:fluttertoast/fluttertoast.dart'; class ToastNotification extends StatefulWidget { const ToastNotification({Key? key}) : super(key: key); @override State<ToastNotification> createState() => _ToastNotificationState(); } class _ToastNotificationState extends State<ToastNotification> { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text('Toast Notification',style: TextStyle(color: Colors.white),), centerTitle: true, backgroundColor: Colors.red, ), body: Center( child: Column( mainAxisAlignment: MainAxisAlignment.center, children: <Widget>[ ElevatedButton( child: Text('Toast Button',style: TextStyle(fontSize: 14,fontWeight: FontWeight.bold,color: Colors.white),), style: ElevatedButton.styleFrom( primary: Colors.green, textStyle: const TextStyle( color: Colors.white, fontSize: 10, fontStyle: FontStyle.normal), ), onPressed: () { Fluttertoast.showToast( msg: "This is Simple Short Toast", ); }, ), ], ), ), ); } }
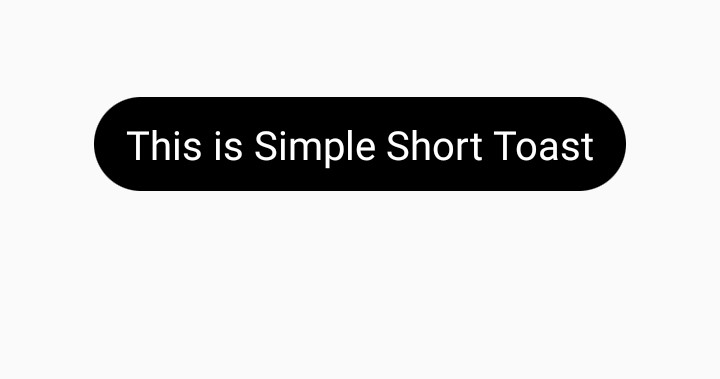
This is a simple Toast Notification you can also customize and add some more properties in it like this
Few properties of Flutter toast:
- toastLength: Toast message duration
- backgroundColor: Background color to be shown
- textColor: Text color to be shown
- fontSize: Toast Message font size
- gravity: Toast message location
1. toastLength
Fluttertoast.showToast( msg: "This is Simple Short Toast", toastLength: Toast.LENGTH_SHORT, );
2. gravity
Fluttertoast.showToast( msg: "This is Simple Short Toast", toastLength: Toast.LENGTH_SHORT, gravity: ToastGravity.CENTER, );
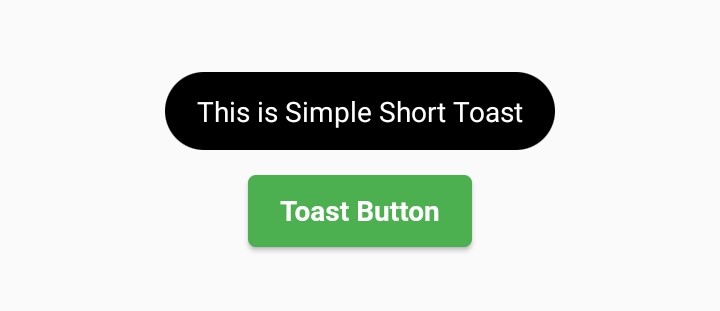
3. fontSize
Fluttertoast.showToast( msg: "Hi Codesinsider !!", toastLength: Toast.LENGTH_LONG, fontSize: 20 );
4. backgroundColor
Fluttertoast.showToast( msg: "Hi Codesinsider !!", toastLength: Toast.LENGTH_LONG, fontSize: 20, backgroundColor: Colors.green );
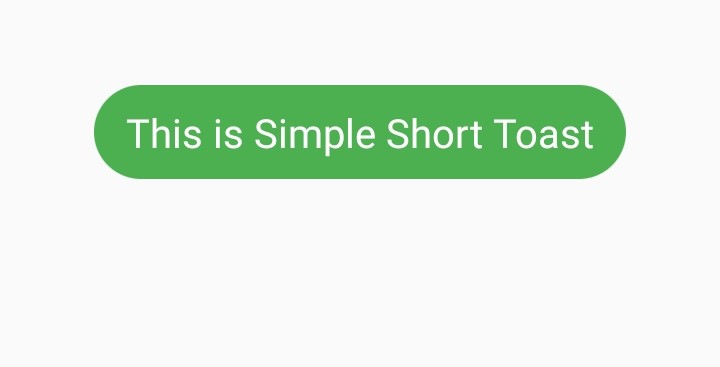
If you want add some attractive Toast Notification in your app then you can add motion_toast 2.6.4 package into your project and add this code in your file like this
import 'package:flutter/foundation.dart'; import 'package:flutter/material.dart'; import 'package:motion_toast/motion_toast.dart'; import 'package:motion_toast/resources/arrays.dart'; class ToastNotification extends StatefulWidget { const ToastNotification({Key? key}) : super(key: key); @override State<ToastNotification> createState() => _ToastNotificationState(); } class _ToastNotificationState extends State<ToastNotification> { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text('Toast Notification',style: TextStyle(color: Colors.white),), centerTitle: true, backgroundColor: Colors.red, ), body: Center( child: SingleChildScrollView( child: Column( mainAxisAlignment: MainAxisAlignment.center, children: <Widget>[ SizedBox( width: 200, child: ElevatedButton( onPressed: () { _displaySuccessMotionToast(); }, child: const Text('Success Motion Toast'), ), ), const SizedBox( height: 10, ), SizedBox( width: 200, child: ElevatedButton( onPressed: () { _displayWarningMotionToast(); }, child: const Text('Warning Motion Toast'), ), ), const SizedBox( height: 10, ), SizedBox( width: 200, child: ElevatedButton( onPressed: () { _displayErrorMotionToast(); }, child: const Text('Error Motion Toast'), ), ), const SizedBox( height: 10, ), SizedBox( width: 200, child: ElevatedButton( onPressed: () { _displayResponsiveMotionToast(); }, child: const Text('Responsive Motion Toast'), ), ), const SizedBox( height: 10, ), SizedBox( width: 200, child: ElevatedButton( onPressed: () { _displaySimultaneouslyToasts(); }, child: const Text('Simultaneously taosts'), ), ), ], ), ) ), ); } void _displaySuccessMotionToast() { MotionToast.success( title: Text( 'Success Motion Toast', style: TextStyle(fontWeight: FontWeight.bold), textAlign: TextAlign.center, ), description: Text( 'this is success message', style: TextStyle(fontSize: 12), textAlign: TextAlign.center, ), layoutOrientation: ToastOrientation.rtl, animationType: AnimationType.fromRight, dismissable: true, ).show(context); } void _displayWarningMotionToast() { MotionToast.warning( title: const Text( 'Warning Motion Toast', style: TextStyle( fontWeight: FontWeight.bold, ), textAlign: TextAlign.center, ), description: Text('This is a Warning',style: TextStyle(fontSize: 12), textAlign: TextAlign.center, ), animationCurve: Curves.bounceIn, borderRadius: 0, animationDuration: const Duration(milliseconds: 1000), ).show(context); } void _displayErrorMotionToast() { MotionToast.error( title: const Text( 'Error', style: TextStyle( fontWeight: FontWeight.bold, ), ), description: const Text('Please enter your name'), position: MotionToastPosition.top, barrierColor: Colors.black.withOpacity(0.3), width: 300, height: 80, dismissable: false, ).show(context); } void _displayResponsiveMotionToast() { MotionToast( icon: Icons.rocket_launch, primaryColor: Colors.purple, title: const Text( 'Custom Toast', style: TextStyle( fontWeight: FontWeight.bold, ), ), description: const Text( 'Hello my name is Flutter dev', ), ).show(context); } void _displaySimultaneouslyToasts() { MotionToast.warning( title: const Text( 'Warning Motion Toast', style: TextStyle( fontWeight: FontWeight.bold, ), ), description: const Text('This is a Warning'), animationCurve: Curves.bounceIn, borderRadius: 0, animationDuration: const Duration(milliseconds: 1000), ).show(context); MotionToast.error( title: const Text( 'Error', style: TextStyle( fontWeight: FontWeight.bold, ), ), description: const Text('Please enter your name'), animationType: AnimationType.fromLeft, position: MotionToastPosition.top, width: 300, height: 80, ).show(context); } }
Output
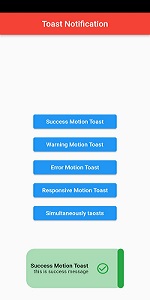
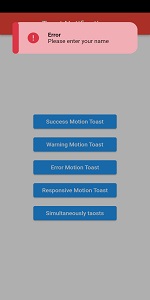
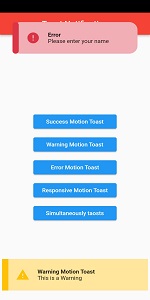
Conclusion
This tutorial shows you how to create a simple toast notification. So If you want to create toast with Build context to have better control over UI. Well, now you know enough about the Flutter toast notification and how to use it in your application. what if I say, now you can add toast notifications to your flutter app with just a click with an app development platform, there is a lot more that helps you to build a Flutter app in minutes.
Thanks for reading this article ❤
If I got something wrong? Let me know in the comments. I would love to improve.
Clap 👏 If this article helps you.
If we got something wrong? Let me know in the comments. we would love to improve.
If you like my work then you also read this article also: How to Implement Showcase view in a flutter, How to implement Pattern lock screen into Flutter, How to create a Scratch Card in flutter using a scratcher package.