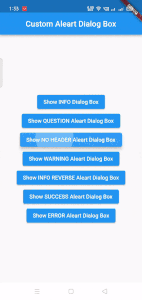
An Alert Dialog box is mainly used when the user exit the app or when deleting some data it confirms by the user you actually want this process. so in this article we learn how to create and implement an alert dialog box in flutter. Alert box informs the user about the situation that requires acknowledgment In this article we learn two ways to implement the first flutter in-build AlertDialog function that we will use like AlertDialog() and the other method is add awesome_dialog 3.0.2 package. awesome_dialog creates attractive and many different-different types of fully custom dialog boxes. you learn all custom dialog boxes and create and implement the best and so easy method.
Step 1: First we create a simple flutter in-build AleartDialog Box
import 'package:flutter/material.dart';
class AleartDialogBox extends StatefulWidget {
const AleartDialogBox({Key? key}) : super(key: key);
@override
State<AleartDialogBox> createState() => _AleartDialogBoxState();
}
class _AleartDialogBoxState extends State<AleartDialogBox> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
centerTitle: true,
title: Text('Aleart Dialog Box'),
),
body: Center(
child: ElevatedButton(
onPressed: (){
showDialog(
context: context,
builder: (ctx) => AlertDialog(
title: const Text("Alert Dialog Box"),
content: const Text("This is simple alert dialog box"),
actions: <Widget>[
TextButton(
onPressed: () => Navigator.pop(context, 'Cancel'),
child: const Text('Cancel'),
),
TextButton(
onPressed: () => Navigator.pop(context, 'OK'),
child: const Text('OK'),
),
],
),
);
}, child: Text('Show Aleart Dialog Box'),
),
),
);
}
}
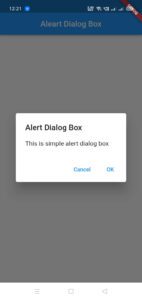
Step 2: import awesome_dialog 3.0.2 package and also the GoogleFont package for font in pubapec.yaml file
dependencies:
awesome_dialog: ^3.0.2
google_fonts: ^3.0.1
Step 3: Now implement this code in your main file for the alert dialog box in a flutter
import 'package:awesome_dialog/awesome_dialog.dart';
import 'package:flutter/material.dart';
import 'package:google_fonts/google_fonts.dart';
class AleartDialogBox extends StatefulWidget {
const AleartDialogBox({Key? key}) : super(key: key);
@override
State<AleartDialogBox> createState() => _AleartDialogBoxState();
}
class _AleartDialogBoxState extends State<AleartDialogBox> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
centerTitle: true,
title: Text('Custom Aleart Dialog Box'),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
ElevatedButton(
onPressed: () {
showAlertinfo(context);
},
child: Text('Show INFO Dialog Box'),
),
ElevatedButton(
onPressed: () {
showAlertquestion(context);
},
child: Text('Show QUESTION Aleart Dialog Box'),
),
ElevatedButton(
onPressed: () {
showAlertnoheader(context);
},
child: Text('Show NO HEADER Aleart Dialog Box'),
),
ElevatedButton(
onPressed: () {
showAlertwarning(context);
},
child: Text('Show WARNING Aleart Dialog Box'),
),
ElevatedButton(
onPressed: () {
showAlertinforeverse(context);
},
child: Text('Show INFO REVERSE Aleart Dialog Box'),
),
ElevatedButton(
onPressed: () {
showAlertsuccess(context);
},
child: Text('Show SUCCESS Aleart Dialog Box'),
),
ElevatedButton(
onPressed: () {
showAlerterror(context);
},
child: Text('Show ERROR Aleart Dialog Box'),
),
],
),
));
}
void showAlertinfo(BuildContext context) {
AwesomeDialog(
context: context,
animType: AnimType.scale,
dialogType: DialogType.info,
dismissOnBackKeyPress: false,
dismissOnTouchOutside: false,
btnOkOnPress: () {},
title: 'This is INFO',
desc: 'this is your description text',
titleTextStyle: GoogleFonts.nunitoSans(
fontSize: 20, fontWeight: FontWeight.bold, color: Colors.black),
descTextStyle: GoogleFonts.nunitoSans(
fontSize: 12, fontWeight: FontWeight.bold, color: Colors.black))
..show();
}
void showAlertquestion(BuildContext context) {
AwesomeDialog(
context: context,
animType: AnimType.scale,
dialogType: DialogType.question,
dismissOnBackKeyPress: false,
dismissOnTouchOutside: false,
btnOkOnPress: () {},
title: 'This is QUESTION',
desc: 'this is your description text',
titleTextStyle: GoogleFonts.nunitoSans(
fontSize: 20, fontWeight: FontWeight.bold, color: Colors.black),
descTextStyle: GoogleFonts.nunitoSans(
fontSize: 12, fontWeight: FontWeight.bold, color: Colors.black))
..show();
}
void showAlertnoheader(BuildContext context) {
AwesomeDialog(
context: context,
animType: AnimType.scale,
dialogType: DialogType.noHeader,
dismissOnBackKeyPress: false,
dismissOnTouchOutside: false,
btnOkOnPress: () {},
title: 'This is NO HEADER',
desc: 'this is your description text',
titleTextStyle: GoogleFonts.nunitoSans(
fontSize: 20, fontWeight: FontWeight.bold, color: Colors.black),
descTextStyle: GoogleFonts.nunitoSans(
fontSize: 12, fontWeight: FontWeight.bold, color: Colors.black))
..show();
}
void showAlertwarning(BuildContext context) {
AwesomeDialog(
context: context,
animType: AnimType.scale,
dialogType: DialogType.warning,
dismissOnBackKeyPress: false,
dismissOnTouchOutside: false,
btnOkOnPress: () {},
title: 'This is WARNING',
desc: 'this is your description text',
titleTextStyle: GoogleFonts.nunitoSans(
fontSize: 20, fontWeight: FontWeight.bold, color: Colors.black),
descTextStyle: GoogleFonts.nunitoSans(
fontSize: 12, fontWeight: FontWeight.bold, color: Colors.black))
..show();
}
void showAlertinforeverse(BuildContext context) {
AwesomeDialog(
context: context,
animType: AnimType.scale,
dialogType: DialogType.infoReverse,
dismissOnBackKeyPress: false,
dismissOnTouchOutside: false,
btnOkOnPress: () {},
title: 'This is INFO REVERSE',
desc: 'this is your description text',
titleTextStyle: GoogleFonts.nunitoSans(
fontSize: 20, fontWeight: FontWeight.bold, color: Colors.black),
descTextStyle: GoogleFonts.nunitoSans(
fontSize: 12, fontWeight: FontWeight.bold, color: Colors.black))
..show();
}
void showAlertsuccess(BuildContext context) {
AwesomeDialog(
context: context,
animType: AnimType.scale,
dialogType: DialogType.success,
dismissOnBackKeyPress: false,
dismissOnTouchOutside: false,
btnOkOnPress: () {},
title: 'This is SUCCESS',
desc: 'this is your description text',
titleTextStyle: GoogleFonts.nunitoSans(
fontSize: 20, fontWeight: FontWeight.bold, color: Colors.green),
descTextStyle: GoogleFonts.nunitoSans(
fontSize: 12, fontWeight: FontWeight.bold, color: Colors.black))
..show();
}
void showAlerterror(BuildContext context) {
AwesomeDialog(
context: context,
animType: AnimType.scale,
dialogType: DialogType.error,
dismissOnBackKeyPress: false,
dismissOnTouchOutside: false,
btnOkOnPress: () {},
title: 'This is ERROR',
desc: 'this is your description text',
titleTextStyle: GoogleFonts.nunitoSans(
fontSize: 20, fontWeight: FontWeight.bold, color: Colors.red),
descTextStyle: GoogleFonts.nunitoSans(
fontSize: 12, fontWeight: FontWeight.bold, color: Colors.black))
..show();
}
}