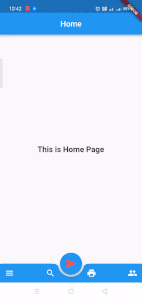
First, we understand what is Bottom Navigation bar; is and how to include Bottom Navigation Bar on Flutter App.The bottom navigation bar consists of multiple items in the form of text labels. icons or both laid out on top of a piece of material. It provides quick navigation between the top-level views of an app. for a larger screen, side navigation may be a better fit. A bottom navigation bar is typically used in conjunction with a scaffold, where it is provided as the Scaffold. bottom navigation bar argument. The type of the bottom navigation bar changes how its items are displayed. If not specified, it is automatically set to the type Bottom Navigation Bar. When there are less than four items and the BottomNavigationBar type. otherwise shifting. In this guide, we are going to create and show the best way to set a Bottom Navigation Bar on Flutter App. this bottom bar makes your app-to-user interface beautiful. so connect with us and follow our all steps
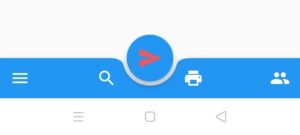
Step 1: Add Bottom Navigation Bar on Flutter App you must create a class name BoTTomNavigationbar.dart
import 'package:flutter/material.dart';
import 'package:navigationdrawer/Navigation3.dart';
import 'package:navigationdrawer/Navigation4.dart';
import 'package:navigationdrawer/navigation1.dart';
import 'Navigation2.dart';
class BoTTomNavigationbar extends StatefulWidget {
const BoTTomNavigationbar({Key? key}) : super(key: key);
@override
State<BoTTomNavigationbar> createState() => _BoTTomNavigationbar();
}
class _BoTTomNavigationbar extends State<BoTTomNavigationbar> {
@override
Widget build(BuildContext context) {
return
Scaffold(
appBar: AppBar(
centerTitle: true,
automaticallyImplyLeading: false,
title: Text('Home'),
),
floatingActionButton:FloatingActionButton( //Floating action button on Scaffold
onPressed: (){
//code to execute on button press
},
child: Icon(Icons.send,color: Colors.redAccent,size: 30,), //icon inside button
),
floatingActionButtonLocation: FloatingActionButtonLocation.centerDocked,
//floating action button position to center
bottomNavigationBar: BottomAppBar( //bottom navigation bar on scaffold
color:Colors.blue,
shape: CircularNotchedRectangle(), //shape of notch
notchMargin: 5, //notche margin between floating button and bottom appbar
child: Row( //children inside bottom appbar
mainAxisSize: MainAxisSize.max,
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: <Widget>[
IconButton(icon: Icon(Icons.menu, color: Colors.white,), onPressed: () {Navigator.push(context, MaterialPageRoute(builder: (context)=>Navigation1()));},),
IconButton(icon: Icon(Icons.search, color: Colors.white,), onPressed: () {Navigator.push(context, MaterialPageRoute(builder: (context)=>Navigation2()));}),
IconButton(icon: Icon(Icons.print, color: Colors.white,), onPressed: () {Navigator.push(context, MaterialPageRoute(builder: (context)=>Navigation3()));},),
IconButton(icon: Icon(Icons.people, color: Colors.white,), onPressed: () {Navigator.push(context, MaterialPageRoute(builder: (context)=>Navigation4()));},),
],
),
),
body: Center(child: Text('This is Home Page',style: TextStyle(fontSize: 20,fontWeight: FontWeight.bold),),)
);
}
}
Step 2:Then you create four different pages or classes like this:
Navigation 1
import 'package:flutter/material.dart';
import 'package:navigationdrawer/Navigation2.dart';
import 'package:navigationdrawer/Navigation3.dart';
import 'package:navigationdrawer/Navigation4.dart';
import 'BottomNavigationBar.dart';
class Navigation1 extends StatefulWidget {
@override
State<Navigation1> createState() => _Navigation1State();
}
class _Navigation1State extends State<Navigation1> {
@override
Widget build(BuildContext context) {
return
Scaffold(
appBar: AppBar(
centerTitle: true,
automaticallyImplyLeading: false,
title: Text('Navigation1'),
),
floatingActionButton:FloatingActionButton( //Floating action button on Scaffold
onPressed: (){
Navigator.push(context, MaterialPageRoute(builder: (context)=>BoTTomNavigationbar()));
},
child: Icon(Icons.send), //icon inside button
),
floatingActionButtonLocation: FloatingActionButtonLocation.centerDocked,
//floating action button position to center
bottomNavigationBar: BottomAppBar( //bottom navigation bar on scaffold
color:Colors.blue,
shape: CircularNotchedRectangle(), //shape of notch
notchMargin: 5, //notche margin between floating button and bottom appbar
child: Row( //children inside bottom appbar
mainAxisSize: MainAxisSize.max,
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: <Widget>[
IconButton(icon: Icon(Icons.menu, color: Colors.redAccent,), onPressed: () {},iconSize: 30,),
IconButton(icon: Icon(Icons.search, color: Colors.white,), onPressed: () {Navigator.push(context, MaterialPageRoute(builder: (context)=>Navigation2()));},),
IconButton(icon: Icon(Icons.print, color: Colors.white,), onPressed: () {Navigator.push(context, MaterialPageRoute(builder: (context)=>Navigation3()));},),
IconButton(icon: Icon(Icons.people, color: Colors.white,), onPressed: () {Navigator.push(context, MaterialPageRoute(builder: (context)=>Navigation4()));},),
],
),
),
body: Center(child: Text('This is Novigation 1',style: TextStyle(fontSize: 18,fontWeight: FontWeight.bold),),)
);
}
}
Navigation 2
import 'package:flutter/material.dart';
import 'package:navigationdrawer/Navigation3.dart';
import 'package:navigationdrawer/Navigation4.dart';
import 'package:navigationdrawer/navigation1.dart';
import 'BottomNavigationBar.dart';
class Navigation2 extends StatefulWidget {
@override
State<Navigation2> createState() => _Navigation2State();
}
class _Navigation2State extends State<Navigation2> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
centerTitle: true,
automaticallyImplyLeading: false,
title: Text('Navigation2'),
),
floatingActionButton:FloatingActionButton( //Floating action button on Scaffold
onPressed: (){
Navigator.push(context, MaterialPageRoute(builder: (context)=>BoTTomNavigationbar()));
},
child: Icon(Icons.send), //icon inside button
),
floatingActionButtonLocation: FloatingActionButtonLocation.centerDocked,
//floating action button position to center
bottomNavigationBar: BottomAppBar( //bottom navigation bar on scaffold
color:Colors.blue,
shape: CircularNotchedRectangle(), //shape of notch
notchMargin: 5, //notche margin between floating button and bottom appbar
child: Row( //children inside bottom appbar
mainAxisSize: MainAxisSize.max,
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: <Widget>[
IconButton(icon: Icon(Icons.menu, color: Colors.white,), onPressed: () {Navigator.push(context, MaterialPageRoute(builder: (context)=>Navigation1()));},),
IconButton(icon: Icon(Icons.search, color: Colors.redAccent,), onPressed: (){},iconSize: 30,),
IconButton(icon: Icon(Icons.print, color: Colors.white,), onPressed: () {Navigator.push(context, MaterialPageRoute(builder: (context)=>Navigation3()));}),
IconButton(icon: Icon(Icons.people, color: Colors.white,), onPressed: () {Navigator.push(context, MaterialPageRoute(builder: (context)=>Navigation4()));},),
],
),
),
body: Center(child: Text('This is Novigation 2',style: TextStyle(fontSize: 18,fontWeight: FontWeight.bold),),)
);
}
}
Navigation 3
import 'package:flutter/material.dart';
import 'package:navigationdrawer/Navigation2.dart';
import 'package:navigationdrawer/Navigation4.dart';
import 'package:navigationdrawer/navigation1.dart';
import 'BottomNavigationBar.dart';
class Navigation3 extends StatefulWidget {
@override
State<Navigation3> createState() => _Navigation3State();
}
class _Navigation3State extends State<Navigation3> {
@override
Widget build(BuildContext context) {
return
Scaffold(
appBar: AppBar(
centerTitle: true,
automaticallyImplyLeading: false,
title: Text('Navigation3'),
),
floatingActionButton:FloatingActionButton( //Floating action button on Scaffold
onPressed: (){
Navigator.push(context, MaterialPageRoute(builder: (context)=>BoTTomNavigationbar()));
},
child: Icon(Icons.send), //icon inside button
),
floatingActionButtonLocation: FloatingActionButtonLocation.centerDocked,
//floating action button position to center
bottomNavigationBar: BottomAppBar( //bottom navigation bar on scaffold
color:Colors.blue,
shape: CircularNotchedRectangle(), //shape of notch
notchMargin: 5, //notche margin between floating button and bottom appbar
child: Row( //children inside bottom appbar
mainAxisSize: MainAxisSize.max,
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: <Widget>[
IconButton(icon: Icon(Icons.menu, color: Colors.white,), onPressed: () {Navigator.push(context, MaterialPageRoute(builder: (context)=>Navigation1()));},),
IconButton(icon: Icon(Icons.search, color: Colors.white,), onPressed: () {Navigator.push(context, MaterialPageRoute(builder: (context)=>Navigation2()));},),
IconButton(icon: Icon(Icons.print, color: Colors.redAccent,), onPressed: () {},iconSize: 30,),
IconButton(icon: Icon(Icons.people, color: Colors.white,), onPressed: () {Navigator.push(context, MaterialPageRoute(builder: (context)=>Navigation4()));}),
],
),
),
body: Center(child: Text('This is Novigation 3',style: TextStyle(fontSize: 18,fontWeight: FontWeight.bold),),)
);
}
}
Navigation 4
import 'package:flutter/material.dart';
import 'package:navigationdrawer/Navigation2.dart';
import 'package:navigationdrawer/Navigation3.dart';
import 'package:navigationdrawer/navigation1.dart';
import 'BottomNavigationBar.dart';
class Navigation4 extends StatefulWidget {
@override
State<Navigation4> createState() => _Navigation4State();
}
class _Navigation4State extends State<Navigation4> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
centerTitle: true,
automaticallyImplyLeading: false,
title: Text('Navigation4'),
),
floatingActionButton:FloatingActionButton( //Floating action button on Scaffold
onPressed: (){
Navigator.push(context, MaterialPageRoute(builder: (context)=>BoTTomNavigationbar()));
},
child: Icon(Icons.send), //icon inside button
),
floatingActionButtonLocation: FloatingActionButtonLocation.centerDocked,
//floating action button position to center
bottomNavigationBar: BottomAppBar( //bottom navigation bar on scaffold
color:Colors.blue,
shape: CircularNotchedRectangle(), //shape of notch
notchMargin: 5, //notche margin between floating button and bottom appbar
child: Row( //children inside bottom appbar
mainAxisSize: MainAxisSize.max,
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: <Widget>[
IconButton(icon: Icon(Icons.menu, color: Colors.white,), onPressed: () {Navigator.push(context, MaterialPageRoute(builder: (context)=>Navigation1()));},),
IconButton(icon: Icon(Icons.search, color: Colors.white,), onPressed: () {Navigator.push(context, MaterialPageRoute(builder: (context)=>Navigation2()));},),
IconButton(icon: Icon(Icons.print, color: Colors.white,), onPressed: () {Navigator.push(context, MaterialPageRoute(builder: (context)=>Navigation3()));}),
IconButton(icon: Icon(Icons.people, color: Colors.redAccent,), onPressed: () {},iconSize: 30,),
],
),
),
body: Center(child: Text('This is Novigation 4',style: TextStyle(fontSize: 18,fontWeight: FontWeight.bold),),
),
);
}
}
Output
Conclusion
In this article, we learn and create a Bottom Navigation Bar on Flutter App in new and attractive ways. the process of creating first we create a new class name BoTTomNavigationbar.dart and add some code there then we create four pages in which user shuffle easily and the name is like Notification1, Notification2, Notification3, and Notification4.
For the navigation the use we are using methods like Navigator.push(context, MaterialPageRoute(builder: (context)=>BoTTomNavigationbar()));
In this flutter, method users shuffle easily with our all pages. So thanks for your passion for stay with us you can also see and learn more useful article on this site.