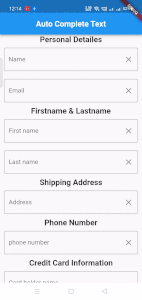
Hii guys today we learned how to create Autocomplete TextField & Autofill Services through simple steps. AutofillHints property is a list of strings that help the autofill service identify the type of this text input. When set to null, this text input will not send its autofill information to the platform, preventing it from participating in autofill triggered by a different AutofillClient, even if they’re in the same AutofillScope. Additionally, on Android and the web, setting this to null will disable autofill for this text field.
Setting up iOS autofill:
To provide the best user experience and ensure your app fully supports password autofill on iOS, follow these steps:
- Set up your iOS app’s associated domains.
- Some autofill hints only work with specific keyboardTypes. For example, AutofillHints.name requires TextInputType.name, and AutofillHints.email works only with TextInputType.emailAddress. Make sure the input field has a compatible keyboardType. Empirically, TextInputType.name works well with many autofill hints that are predefined on iOS.
we create the form which fills personal details with Autocomplete TextField & Autofill Services.
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
class AutoCompleteText extends StatefulWidget {
const AutoCompleteText({Key? key}) : super(key: key);
@override
State<AutoCompleteText> createState() => _AutoCompleteTextState();
}
class _AutoCompleteTextState extends State<AutoCompleteText> {
final NameController = TextEditingController();
final EmailController = TextEditingController();
final FirstNameController = TextEditingController();
final LastNameController = TextEditingController();
final AddressController = TextEditingController();
final PhoneNumberController = TextEditingController();
@override
Widget build(BuildContext context) {
final String title = "Auto Complete Text";
final style = TextStyle(fontSize: 20,fontWeight: FontWeight.bold);
return Scaffold(
appBar: AppBar(
centerTitle: true,
title: Text(
title,
style: style,
),
),
body: SingleChildScrollView(
child: Column(
children: <Widget>[
Text('Personal Detailes',style: TextStyle(fontSize: 20,fontWeight: FontWeight.bold),),
Padding(
padding: EdgeInsets.all(10),
child: TextField(
controller: NameController,
decoration: InputDecoration(
labelText: 'Name',
border: OutlineInputBorder(),suffixIcon: IconButton(
onPressed: NameController.clear,
icon: Icon(Icons.clear),
),),
keyboardType: TextInputType.name,
autofillHints: [AutofillHints.name],
),
),
Padding(
padding: EdgeInsets.all(10),
child: TextField(
controller: EmailController,
decoration: InputDecoration(
labelText: 'Email',
border: OutlineInputBorder(),suffixIcon: IconButton(
onPressed: EmailController.clear,
icon: Icon(Icons.clear),
),),
keyboardType: TextInputType.emailAddress,
autofillHints: [AutofillHints.email],
),
),
Text('Firstname & Lastname',style: TextStyle(fontSize: 20,fontWeight: FontWeight.bold),),
AutofillGroup(
child: Column(
children: <Widget>[
Padding(
padding: EdgeInsets.all(10),
child: TextField(
controller: FirstNameController,
decoration: InputDecoration(
labelText: 'First name',
border: OutlineInputBorder(),suffixIcon: IconButton(
onPressed: FirstNameController.clear,
icon: Icon(Icons.clear),
),),
keyboardType: TextInputType.name,
autofillHints: [AutofillHints.givenName],
),
),
Padding(
padding: EdgeInsets.all(10),
child: TextField(
controller: LastNameController,
decoration: InputDecoration(
labelText: 'Last name',
border: OutlineInputBorder(),suffixIcon: IconButton(
onPressed: LastNameController.clear,
icon: Icon(Icons.clear),
),),
keyboardType: TextInputType.name,
autofillHints: [AutofillHints.familyName],
),
),
],
),
),
Text('Shipping Address',style: TextStyle(fontSize: 20,fontWeight: FontWeight.bold),),
Padding(
padding: EdgeInsets.all(10),
child: TextField(
controller: AddressController,
decoration: InputDecoration(
labelText: 'Address',
border: OutlineInputBorder(),suffixIcon: IconButton(
onPressed: AddressController.clear,
icon: Icon(Icons.clear),
),),
keyboardType: TextInputType.streetAddress,
autofillHints: [AutofillHints.postalAddress],
),
),
Text('Phone Number',style: TextStyle(fontSize: 20,fontWeight: FontWeight.bold),),
Padding(
padding: EdgeInsets.all(10),
child: TextField(
controller: PhoneNumberController,
decoration: InputDecoration(
labelText: 'phone number',
border: OutlineInputBorder(),suffixIcon: IconButton(
onPressed: PhoneNumberController.clear,
icon: Icon(Icons.clear),
),),
keyboardType: TextInputType.phone,
autofillHints: [AutofillHints.telephoneNumberNational],
),
),
],
),
),
);
}
}
this time we create fill-in Credit card information.
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
class AutoCompleteText extends StatefulWidget {
const AutoCompleteText({Key? key}) : super(key: key);
@override
State<AutoCompleteText> createState() => _AutoCompleteTextState();
}
class _AutoCompleteTextState extends State<AutoCompleteText> {
@override
Widget build(BuildContext context) {
final String title = "Auto Complete Text";
final style = TextStyle(fontSize: 20,fontWeight: FontWeight.bold);
return Scaffold(
appBar: AppBar(
centerTitle: true,
title: Text(
title,
style: style,
),
),
body: SingleChildScrollView(
child: Column(
children: <Widget>[
Text('Credit Card Information',style: TextStyle(fontSize: 20,fontWeight: FontWeight.bold),),
AutofillGroup(
child: Column(
children: <Widget>[
Padding(
padding: EdgeInsets.all(10),
child: TextField(
decoration: InputDecoration(
labelText: 'Card holder name',
border: OutlineInputBorder(),
),
keyboardType: TextInputType.name,
autofillHints: [AutofillHints.creditCardName],
),
),
Padding(
padding: EdgeInsets.all(10),
child: TextField(
decoration: InputDecoration(
labelText: 'Card number',
border: OutlineInputBorder(),
),
keyboardType: TextInputType.number,
autofillHints: [AutofillHints.creditCardNumber],
),
),
Padding(
padding: EdgeInsets.all(10),
child: TextField(
decoration: InputDecoration(
labelText: 'MM/YY',
border: OutlineInputBorder(),
),
autofillHints: [AutofillHints.creditCardExpirationDate],
),
),
Padding(
padding: EdgeInsets.all(10),
child: TextField(
decoration: InputDecoration(
labelText: 'Security Code',
border: OutlineInputBorder(),
),
obscureText: true,
keyboardType: TextInputType.visiblePassword,
autofillHints: [AutofillHints.creditCardSecurityCode],
),
),
],
),
),
Text('UserName & Password',style: TextStyle(fontSize: 20,fontWeight: FontWeight.bold),),
AutofillGroup(
child: Column(
children: <Widget>[
Padding(
padding: EdgeInsets.all(10),
child: TextField(
decoration: InputDecoration(
labelText: 'User name',
border: OutlineInputBorder(),
),
keyboardType: TextInputType.name,
textInputAction: TextInputAction.next,
autofillHints: [AutofillHints.username],
),
),
Padding(
padding: EdgeInsets.all(10),
child: TextField(
decoration: InputDecoration(
labelText: 'Password',
border: OutlineInputBorder(),
),
obscureText: true,
keyboardType: TextInputType.visiblePassword,
autofillHints: [AutofillHints.password],
),
),
],
),
),
Padding(
padding: EdgeInsets.all(10),
child: ElevatedButton(
child: Text('Submit',style: TextStyle(fontSize: 18),),
style: ElevatedButton.styleFrom(
primary: Colors.green,
minimumSize: const Size.fromHeight(50),),
onPressed: () {
Navigator.push(context, MaterialPageRoute(builder: (context)=>FlutterTypeAhead()));
},
),
),
],
),
),
);
}
}
Last but not the least we fill Username & Password
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
class AutoCompleteText extends StatefulWidget {
const AutoCompleteText({Key? key}) : super(key: key);
@override
State<AutoCompleteText> createState() => _AutoCompleteTextState();
}
class _AutoCompleteTextState extends State<AutoCompleteText> {
@override
Widget build(BuildContext context) {
final String title = "Auto Complete Text";
final style = TextStyle(fontSize: 20,fontWeight: FontWeight.bold);
return Scaffold(
appBar: AppBar(
centerTitle: true,
title: Text(
title,
style: style,
),
),
body: SingleChildScrollView(
child: Column(
children: <Widget>[
Text('UserName & Password',style: TextStyle(fontSize: 20,fontWeight: FontWeight.bold),),
AutofillGroup(
child: Column(
children: <Widget>[
Padding(
padding: EdgeInsets.all(10),
child: TextField(
decoration: InputDecoration(
labelText: 'User name',
border: OutlineInputBorder(),
),
keyboardType: TextInputType.name,
textInputAction: TextInputAction.next,
autofillHints: [AutofillHints.username],
),
),
Padding(
padding: EdgeInsets.all(10),
child: TextField(
decoration: InputDecoration(
labelText: 'Password',
border: OutlineInputBorder(),
),
obscureText: true,
keyboardType: TextInputType.visiblePassword,
autofillHints: [AutofillHints.password],
),
),
],
),
),
],
),
),
);
}
}