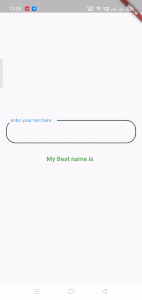
The state of the app can be defined very simply as the one that is present in the app’s memory while the app is running. It includes all the widgets that maintain the UI of the app including buttons, text fonts, icons, animations, etc. So now as we know what these states are, let’s dive straight into our main topic i.e. what are these stateful widgets and stateless widgets and how do they differ from each other?
State: State is the information that can be synchronized when the widget is created and can change during the lifetime of the widget.
Stateful vs Stateless Widgets
- When a widget changes(user interact with it) it’s Stateful
CheckBox, RadioButton, Form, Textfield.
- Overrides the createState() and returns a state.
- Use when the UI can change dynamically.
- When the widget’s state changes, the state object calls setState(), telling the framework to redraw the widget.
- No internal state to manage or no direct user interaction, it’s Stateless
Text, RaisedButton, Icon, IconButton
- Overrides the build() and returns a widget.
- Use when the UI depends on the information within the object itself.
Using Stateful Widgets
- Create a class that extends a “Stateful widget”, that returns a State in “createState()”.
- Create a “State” class, with properties that may change.
- Within “State” class, implement the “build)” method.
- Call the setState0 to make the changes. Calling setState() tells framework to redraw widget.
import 'package:flutter/material.dart';
class Statefull extends StatefulWidget {
const Statefull({Key? key}) : super(key: key);
@override
State<Statefull> createState() => _StatefullState();
}
class _StatefullState extends State<Statefull> {
String name = "";
@override
Widget build(BuildContext context) {
return Padding(
padding: EdgeInsets.all(15),
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
crossAxisAlignment: CrossAxisAlignment.center,
children: <Widget>[
TextField(
decoration: InputDecoration(
labelText: 'enter your text here...',
fillColor: Colors.white,
focusedBorder:OutlineInputBorder(
borderSide: const BorderSide(color: Colors.black, width: 2.0),
borderRadius: BorderRadius.circular(25.0),
),
border: OutlineInputBorder(),
),
keyboardType: TextInputType.text,
textInputAction: TextInputAction.done,
onChanged: (String string){
setState(() {
name = string;
});
},
onSubmitted: (String string){
setState(() {
name = string;
});
},
),
SizedBox(
height: 30,
),
Text('My Best name is $name',style: TextStyle(fontSize: 16,fontWeight: FontWeight.bold,color: Colors.green),),
],
),
);
}
}
Output
Stateless Widgets: Widgets whose state once created cannot be changed, are called stateless widgets. Once created these widgets become immutable i.e. any change in the variable, icon, button or retrieve data cannot change the state of the app. The basic structure of a stateless widget is given below. Stateless Widgets override the build() method and return a Widget. For example, we use text or icon is our flutter application where the position of the widget does not change at runtime. It is used when the UI relies on information within the object itself. Other examples can be text, raised button, iconbutton.
import 'package:flutter/material.dart';
class Stateless extends StatelessWidget {
const Stateless({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
centerTitle: true,
title: Text('Stateless Widgets',style: TextStyle(fontSize: 20, fontWeight: FontWeight.bold),),
),
body: Center(
child: Text('This is Stateless widgets',style: TextStyle(color: Colors.green,fontWeight: FontWeight.bold,fontSize: 16),),
),
);
}
}