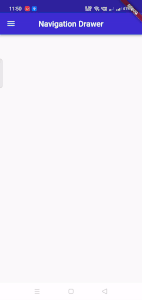
how to add a navigation drawer in flutter The navigation drawer in Flutter allows users to navigate to different pages in your app. The navigation drawer is added using the drawer widget. It can be opened via swipe gesture or by clicking on the menu icon in the app bar.
Typically, the navigation drawer opens from the left side of the screen, but you can also configure it to open from the right side (for LTR text settings). When it opens, the drawer covers about 60-70 percent of the screen, and to close it, you can simply swipe or click outside the drawer.
In this tutorial, we’ll learn how to add the navigation drawer in Flutter and how to customize it. Here’s what we’ll cover:
- When to use a navigation drawer.
- How to add a basic navigation drawer in Flutter
- Displaying user details in navigation drawer header
- Displaying About ListTile Opening a navigation drawer programmatically
- Opening a navigation drawer from the right side
- Controlling navigation drawer width
Step 1: Crete a new project in flutter and edit the main.dart file
import 'package:flutter/material.dart';
import 'HomePage.dart';
void main() {
runApp(MainApp());
}
class MainApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: HomePage(),
);
}
}
Step 2: Create a new file in the lib folder name HomePage.dart
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
class HomePage extends StatelessWidget {
final padding = EdgeInsets.symmetric(horizontal: 20);
final String home = 'Home';
final String page1 = 'Page1';
final String page2 = 'Page2';
final urlImage = 'https://images.unsplash.com/photo-1494790108377-be9c29b29330?ixlib=rb-4.0.3&ixid=MnwxMjA3fDB8MHxzZWFyY2h8MXx8dXNlciUyMHByb2ZpbGV8ZW58MHx8MHx8&w=1000&q=80';
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
centerTitle: true,
title: Text('Navigation Drawer'),
backgroundColor: const Color(0xff3f2dd2),
),
drawer: Drawer(
child: Material(
color: Color.fromRGBO(50, 75, 205, 1),
child: ListView(
children: <Widget>[
buildHeader(
urlImage: urlImage,
onClicked: (){
//code after click function...
},
),
Container(
padding: padding,
child: Column(
children: [
Divider(color: Colors.white70),
const SizedBox(height: 10),
GestureDetector(
onTap: (){
//code after click...
},
child: buildMenuItem(
text: home,
icon: Icons.home,
),
),
const SizedBox(height: 10),
GestureDetector(
onTap: (){
//code after click...
},
child: buildMenuItem(
text: page1,
icon: Icons.assignment,
),
),
const SizedBox(height: 10),
GestureDetector(
onTap: (){
//code after click...
},
child: buildMenuItem(
text: page2,
icon: Icons.badge,
),
),
const SizedBox(height: 10),
],
),
),
],
),
),
),
);
}
Widget buildHeader({
required String urlImage,
required VoidCallback onClicked,
}) => InkWell(
onTap: onClicked,
child: SingleChildScrollView(
child: Container(
padding: padding.add(EdgeInsets.symmetric(vertical: 40)),
child: Row(
children: [
CircleAvatar(radius: 20, backgroundImage: NetworkImage(urlImage)),
SizedBox(width: 20),
Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Text(
'User Name',
style: TextStyle(fontSize: 16, color: Colors.white),
),
const SizedBox(height: 4,),
Text(
'[email protected]',
style: TextStyle(fontSize: 12, color: Colors.white),
),
const SizedBox(height: 4,),
Text(
'+9199988xxxxx',
style: TextStyle(fontSize: 12, color: Colors.white),
),
],
),
Spacer(),
CircleAvatar(
radius: 20,
backgroundColor: Color.fromRGBO(30, 60, 168, 1),
child: Icon(Icons.edit, color: Colors.white),
)
],
),
),
),
);
Widget buildMenuItem({
required String text,
required IconData icon,
VoidCallback? onClicked,
}) {
final color = Colors.white;
final hoverColor = Colors.white70;
return ListTile(
leading: Icon(icon, color: color),
title: Text(text, style: TextStyle(color: color)),
hoverColor: hoverColor,
onTap: onClicked,
);
}
}